Your First Script
In this lesson, it's time to write our first PHP script.
Understanding the PHP interpreter
Our journey begins by understanding how the PHP interpreter works. The PHP interpreter is a program that processes PHP code and generates the output that is sent to the client's web browser.
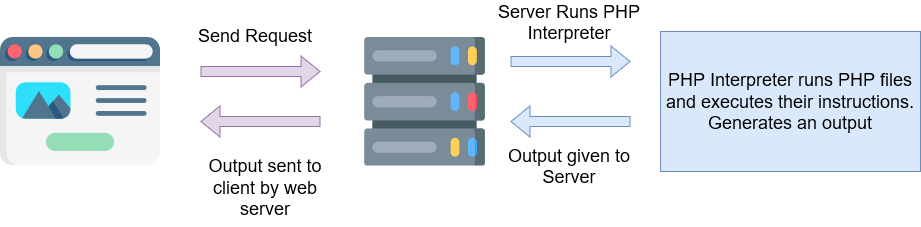
When a user requests a PHP page, the following steps take place:
- A browser initiates a request to a web server.
- The web server receives the request and passes it to the PHP interpreter.
- The PHP interpreter reads the PHP code from the requested file and converts it into a series of instructions that can be executed by the computer.
- The PHP interpreter interacts with any databases or other resources as necessary to retrieve or store data based on the instructions in the PHP code.
- The PHP interpreter generates the output, which is typically HTML and CSS and sends it back to the web server.
- The web server sends the output back to the client's web browser, which displays the result to the user, which is typically an HTML page.
Think of the PHP interpreter as a chef in a kitchen who takes ingredients and follows a recipe to make a dish. Just like a chef takes the ingredients and follows the instructions in a recipe to create a meal, the PHP interpreter takes the PHP code and follows the instructions to generate the output that is sent to the user's web browser.
Using XAMPP
Luckily, by using XAMPP, we do not need to install or configure a web server and PHP. They both have been prepared by XAMPP and are ready to go! On your system, the XAMPP Control Panel program should be installed on your machine. Open it.
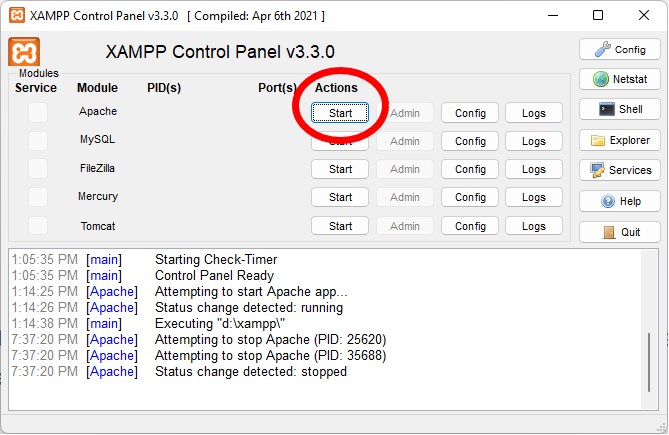
In the image above, there is a list of services that can turn on with a click of a button. For the time being, we only need to turn on Apache. So, press the Start button as highlighted in the image.
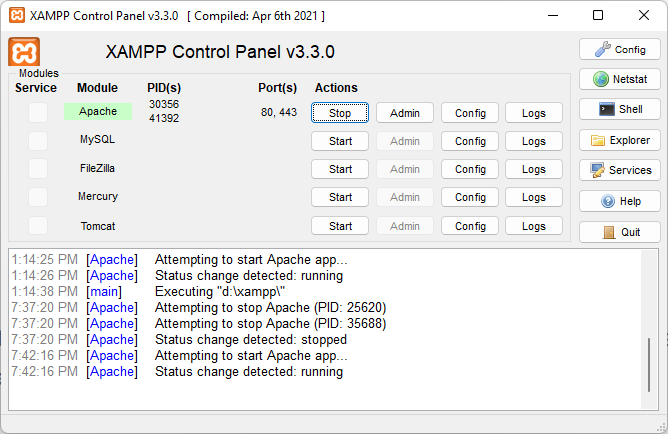
What about PHP?
Apache is a program that can be used to create a web server. It'll handle intercepting requests and sending back responses, which is typically an HTML page generated by PHP.
By turning on Apache, it'll handle giving the request to PHP. Therefore, by turning on Apache, XAMPP also turns on PHP.
The htdocs directory
Now that the server is turned on, we can proceed to create a PHP script. The question is, where? In the XAMPP control panel, there's a button called Explorer. Click on it!
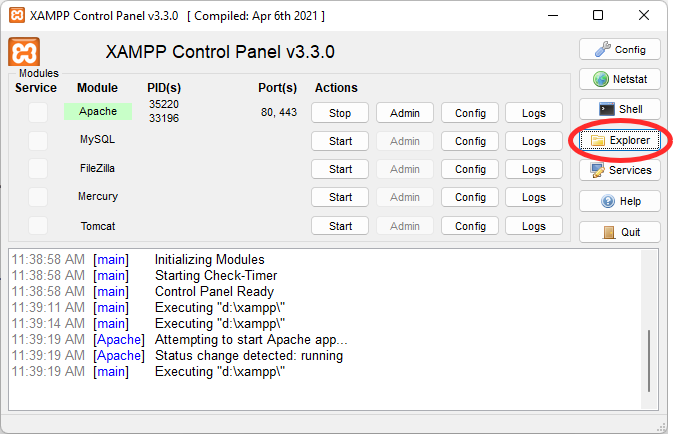
This button opens the file explorer pointing to where XAMPP is installed. Inside this directory, there's a folder called htdocs
. Open this folder.
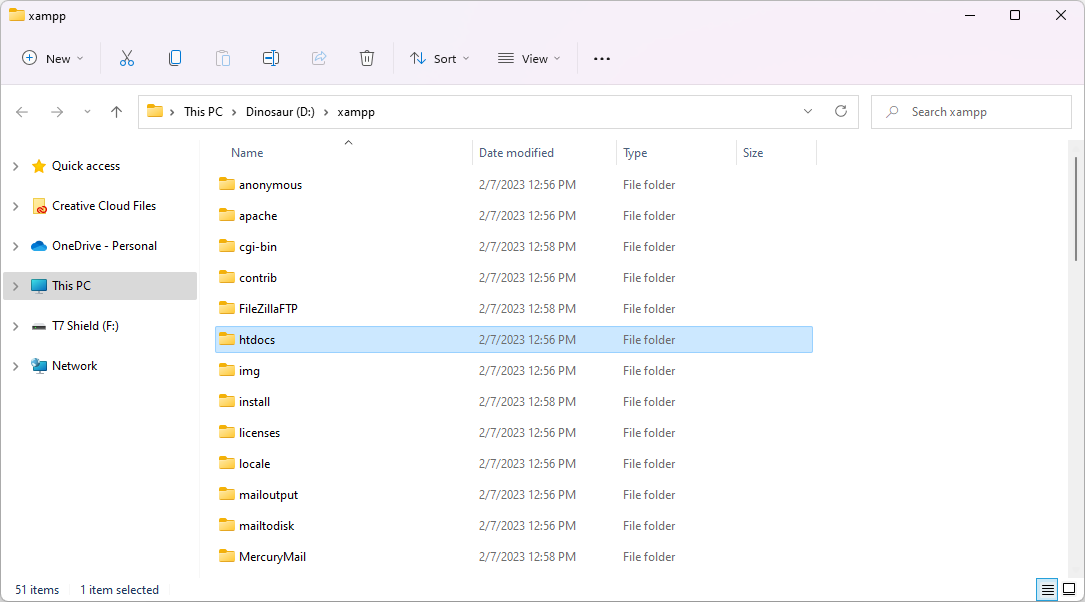
The htdocs
directory in XAMPP is the root directory for the Apache web server. It is where you place your PHP scripts, HTML files, and other resources that you want to serve to clients through the web server.
When you start the Apache server as part of the XAMPP stack, it looks in the htdocs
directory to serve files to clients when they make a request. For example, if a user requests the URL http://localhost/example.php, the Apache server will look for the file "example.php" in the htdocs
directory and serve it to the client.
The htdocs
directory is the starting point for serving content through the Apache web server, and it is an integral part of the XAMPP stack for developing and testing web applications on your local machine.
What is localhost?
In web development, localhost is often used as the hostname for a web server running on your own computer. For example, when you run a web server on your local machine as part of the XAMPP stack, you can access the server and your web applications by entering http://localhost into your web browser.
The term "localhost" is a convenient shorthand for accessing a web server running on the same machine as the client and is often used during development and testing before deploying to a live production environment.
The default installation of XAMPP provides a set of files to demonstrate how files are served to the browser. You can freely delete all these files. I recommend deleting all of them except the index.php
file. We're going to need it.
After deleting the files, you can open the index.php
file with the text editor of your choice. You may see the following:
<?php
if (!empty($_SERVER['HTTPS']) && ('on' == $_SERVER['HTTPS'])) {
$uri = 'https://';
} else {
$uri = 'http://';
}
$uri .= $_SERVER['HTTP_HOST'];
header('Location: '.$uri.'/dashboard/');
exit;
?>
Something is wrong with the XAMPP installation :-(
It's safe to completely empty out the file. We're going to start from scratch.
PHP Pages
A PHP file is a text file that contains PHP code and is saved with a ".php" file extension. If the server detects that the client (browser) is trying to access a PHP file, it'll send the file to the PHP interpreter, generating an output. That output is then sent to the browser.
So, what can we write in a PHP file?
Static text
"Static text" refers to text or content that remains the same across all pages or instances of a web application. It is text that is not dynamically generated based on user input or other dynamic sources.
In a PHP file, you can freely write static text without worrying about breaking anything.
Hello world!
Once you've written random text into the index.php
file, you can view the contents of the file by visiting http://localhost.
How does the server know to serve the index.php file?
By default, if a file is not specified in the URL, the server will
automatically search for an index.html
or index.php
file.
Rendering HTML
As mentioned before, PHP is primarily used for rendering HTML pages. As a result, ALL HTML syntax is supported. HTML documents can be created within a PHP like any other HTML file.
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Hello world</h1>
</body>
</html>
PHP Tags
Out of the box, the PHP interpreter doesn't execute the instructions in our PHP file until we've written a pair of PHP tags. An opening PHP tag is written with <?php
, and the closing PHP tag is written with ?>
.
By writing the opening tag (<?php
), the PHP interpreter will begin executing the code written after it. This process happens before content is sent back to the browser.
On the other hand, writing the closing tag (?>
) causes the interpreter to stop executing code.
<?php ?>
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Hello world</h1>
</body>
</html>
Multiple PHP Tags
We're not limited to a single pair of PHP tags. Multiple tags can be inserted.
<?php ?>
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Hello world</h1>
</body>
</html>
<?php ?>
Mixing PHP and HTML
You can write PHP tags from within HTML tags like so.
<!DOCTYPE html>
<html lang="en">
<body>
<h1><?php ?></h1>
</body>
</html>
Avoid Direct HTML in PHP
You can't write HTML directly inside a pair of PHP tags. That's not to say it's impossible, nor shouldn't you write HTML in PHP. In fact, it's very common.
However, what I am saying is that you can't continue to write HTML inside a pair of PHP tags like you would for any other pair of tags. Otherwise, the PHP interpreter will throw an error at your face for attempting to do so.
So the question becomes, how do you write HTML inside a pair of PHP tags?
<!DOCTYPE html>
<html lang="en">
<body>
<?php <h1></h1> ?>
</body>
</html>
Syntax
Inside PHP tags, we must write valid PHP code. Therefore, we must understand the syntax of PHP.
In PHP, syntax refers to the set of rules that determine how the PHP code should be structured and written. It is like grammar for a language, providing a consistent way for the PHP interpreter to understand and execute the code.
Think of syntax in PHP as the rules for writing a sentence in English. Just like there are rules for the order of words, capitalization, and punctuation, there are rules in PHP for how code is written.
The syntax of PHP code defines the structure and format of the code, and it is crucial to follow the rules of syntax in order for the code to be correct and work as intended. For example, if you forget to put periods at the end of your sentences, it will be difficult to discern where a sentence begins and ends. Can you imagine how difficult reading would be without periods?
Statements
The first step to understanding PHP's syntax is to understand what a statement is. A statement is a single instruction that the PHP interpreter can execute. It is like a sentence in a conversation, where each sentence conveys a specific idea or request.
Just like in a conversation, each statement in PHP has a specific purpose and conveys a specific action. For example, a PHP statement might calculate a total, send an email, or output a message to the browser.
Keywords
Now that we know what syntax and statements are, we can move on to the final piece of the puzzle, which are keywords. Keywords are reserved words that have a special meaning in the language. They are like specific words in a language with a specific meaning and are used for a specific purpose.
Just like in a natural language, keywords in PHP have a set meaning and cannot be redefined. There are many other keywords in PHP, each with a specific purpose and meaning. If you're interested in a complete list of keywords, check out the following documentation page: https://www.php.net/manual/en/reserved.keywords.php
The echo keyword
For this lesson, we're going to focus on the simplest yet most common keyword, which is the echo
keyword.
Using the echo
keyword
The echo
keyword is used to display or output text or data to the web browser. It is like saying something out loud in a conversation, and the text you output is like what you would say out loud.
The echo
keyword is followed by the text or data that you want to output, and the PHP interpreter will send that text to the web browser to be displayed. Messages must be wrapped with a pair of quotes. For example, you could use the following code to display the message "Hello, World!" in the web browser:
<!DOCTYPE html>
<html lang="en">
<body>
<h1><?php echo "Hello, World"; ?></h1>
</body>
</html>
Semicolons
The PHP line of code we've written is considered to be a statement. You may have noticed it, but our statement ends with a ;
character. Statements in PHP are separated by semicolons, and each statement must be written on a separate line.
<!DOCTYPE html>
<html lang="en">
<body>
<h1><?php echo "Hello, World"; ?></h1>
</body>
</html>
Omitting Semicolons
PHP allows you to omit the semicolon if you're writing a single line of PHP code within PHP tags.
It's in the author's opinion that you should always include the ;
character even if you're writing a single line of code. While not required, consistency is always a good habit to build. For the rest of this book, I'll always include the semicolon, even if I'm writing a single line of code.
<!DOCTYPE html>
<html lang="en">
<body>
<h1><?php echo "Hello, World" ?></h1>
</body>
</html>
Multiple Statements
When the PHP interpreter encounters a statement, it executes the instructions contained within it and then moves on to the next statement. By stringing together multiple statements, you can create a PHP script that performs a series of actions.
Here's an example of multiple echo statements. As always, we're ending each statement with a semicolon.
<!DOCTYPE html>
<html lang="en">
<body>
<h1>
<?php
echo "Hello, World";
echo "My name is John.";
echo "I hope you are having a good day.";
?>
</h1>
</body>
</html>
HTML in PHP
Alright, but what about HTML? We can output HTML from within PHP like so.
Unlike before, this is entirely valid PHP. In most PHP applications, this is how HTML gets rendered via PHP. The question is, why would you want to output HTML like this?
This example doesn't demonstrate why you would want to output HTML, just that it's completely possible. In the future, as we learn more PHP features, you'll discover that you can output custom content tailored to each user. By using PHP, we'll be able to swap the message with other messages.
<!DOCTYPE html>
<html lang="en">
<body>
<?php echo "<h1>Hello, World</h1>"; ?>
</body>
</html>
Overall, the echo
keyword is one of the simplest and most common ways to output information in PHP. It is often used in combination with other statements and functions to build dynamic web pages.
One more thing
In some cases, you're not always going to have an HTML document written within a PHP file. If a PHP file is only going to consist of PHP code without HTML, theoretically, you can write your code like this.
<?php
echo "Hello, World!";
?>
However, it's completely acceptable to omit the closing PHP tag (?>
).
<?php
echo "Hello, World!";
If you don't add the closing PHP tag, the PHP interpreter will automatically stop executing instructions at the end of the file. Generally, most developers will omit the closing PHP tag to keep our code readable and more straightforward. In addition, there can be problems caused by leaving the closing PHP tag, which we'll discover as we progress through the book.
Exercise
At the end of most lessons, you'll be given an opportunity to test what you've learned.
For this exercise, write a PHP script that outputs a pair of <p>
tags with a message that says, "My name is John." You must do so with pure PHP.
Key Takeaways
- The PHP interpreter is responsible for executing the instructions/statements written within your PHP files.
- XAMPP serves files from your web server in the
htdocs
folder. - All HTML syntax is valid in PHP.
- We can write PHP code inside a pair of PHP tags, i.e.
<?php ?>
- A statement is a single instruction that the PHP interpreter can execute.
- Keywords are reserved words that have a special meaning in the language.
- The
echo
keyword instructs PHP to output the content written after it. - The closing PHP tag can be omitted if you only plan on writing PHP code inside a PHP file.