Writing Functions Best Practices
As a developer, you'll be writing thousands of functions throughout your career. While there isn't a univeral approach to writing functions, there are some good practices you should be aware of. Consider the following:
- Identify the Purpose: Determine what task the function will perform. The function should have a clear, single purpose to maintain the principle of cohesion.
- Design the Function: Plan the function's inputs (parameters) and output (return value). The inputs should provide all the data the function needs to perform its task, and the output should represent the result of the task.
- Name the Function: The function name should clearly reflect its purpose. Use a verb-noun pair if possible (like
calculateAverage
,printReport
, etc.). - Write the Function Signature: The function signature includes the function name and parameters. The parameters should be well-named and self-explanatory, making the function easy to use without having to look at its implementation.
- Error Handling: Implement appropriate error handling within the function. This can involve checking the validity of input parameters, or catching and handling exceptions as needed.
Following these steps can help ensure that your functions are useful, reliable, and easy to understand and maintain.
Questions to ask
- What is the Purpose of the Function?
Understanding the purpose guides the design and helps ensure the function remains focused on a single task. This follows the principle of single responsibility, leading to cleaner and more maintainable code.
The Principle of Responsibility, often referred to as the Single Responsibility Principle (SRP) in the context of software development, is a principle of computer programming stating that every module, class, or function should have responsibility over a single part of the functionality provided by the software, and that responsibility should be entirely encapsulated by the module, class, or function. This means that each component of a software system should have one, and only one, reason to change.
SRP is one of the five principles of SOLID, an acronym coined by Robert C. Martin, representing a suite of design principles for object-oriented software that is easy to manage, maintain, and scale. Adherence to the Single Responsibility Principle leads to more modular, flexible, and maintainable code. By ensuring each function or class is focused on a specific task, developers can more easily understand the system, isolate issues, and make changes without unexpected side effects. It simplifies unit testing as well, since each function or class has a single behavior to validate.
- What are the Inputs and Outputs?
Clearly defining inputs and outputs helps to understand the data the function requires to perform its task and what it will produce. This ensures the function can be correctly used and integrated with other parts of the code.
- Can the Function be Broken Down Further?
Keeping functions small and focused improves readability and maintainability. If a function is doing multiple things, it may be a sign that it can be broken down into smaller, more manageable pieces.
Should I use functions?
Functions are like the building blocks of a program, helping you organize your code in a neat and efficient manner. Here are some reasons why we use functions:
- Code Reusability: Once a function is defined, it can be used over and over again in your program. This saves you from having to write the same code repeatedly. For example, if you have a piece of code that calculates the average of two numbers, you can put that code in a function and use it wherever you need to calculate an average.
- Code Organization: Functions allow you to break down complex tasks into smaller, manageable parts. Each function performs a specific task. This makes your code easier to read and understand. It's like cooking a complex dish by breaking it down into smaller steps.
- Avoiding Errors: If a piece of code is used in multiple places and needs to be changed, having it in one function means you only need to change it in one place. This can help avoid errors and inconsistencies in your code.
- Ease of Testing and Debugging: Functions can be tested individually which makes finding and fixing errors a lot easier. If something goes wrong, you know the error is likely within the function you're currently working with.
So, you can think of functions like tools in a toolbox. Each tool (function) has a specific job. By using the right tool for the job, you can build something complex (your program) in an organized and efficient way.
PHP Documentation
When reading the documentation for a function, there are several key pieces of information that can help you understand what the function does. Let's look at the str_replace() function:
- Function Name: The function name can often give you a good idea of what the function does. Good function names are descriptive and use a verb-noun pair to describe the action the function performs.
- Description: The description provides a summary of what the function does. It should explain the purpose of the function and give a high-level overview of its behavior.
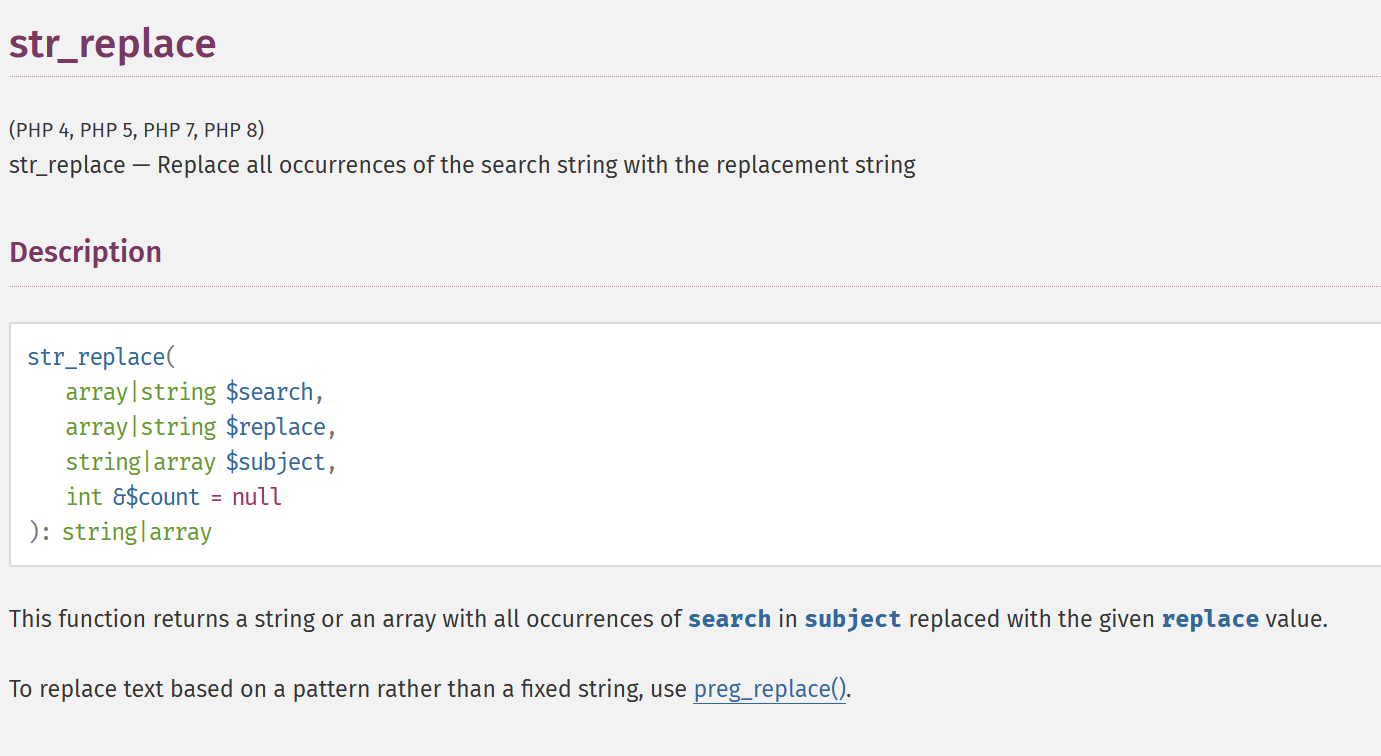
- Parameters: The parameters section lists each input the function can accept, including the type of each parameter and whether it is optional or required. There should also be a brief explanation of what each parameter represents.
- Return Value: The return value section describes the output of the function. It should specify the type of the return value and explain what the return value represents.
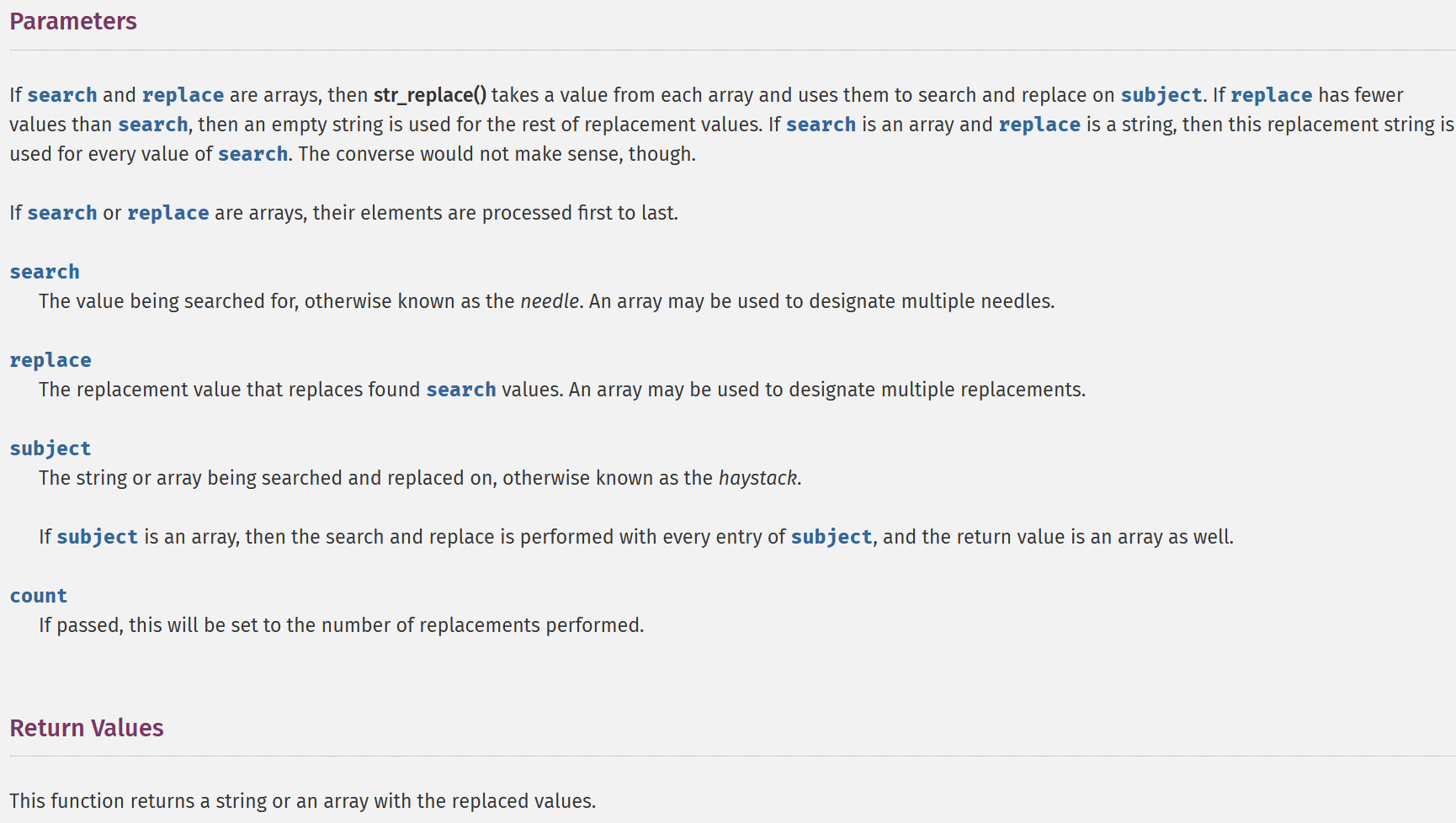
- Examples: Example code can show you how to use the function in a real-world scenario. This can often be the most helpful part of the documentation, as it gives you a practical understanding of how the function works.
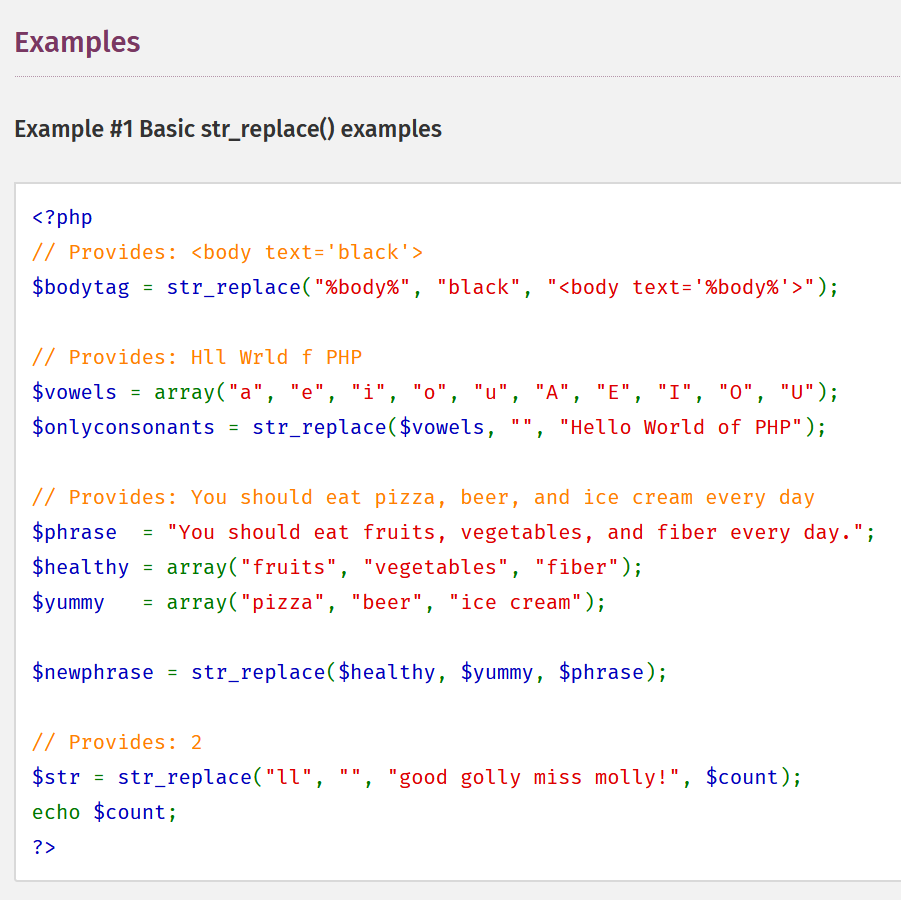
- See Also: This section might reference related functions or further reading that can help you understand the function in a broader context.
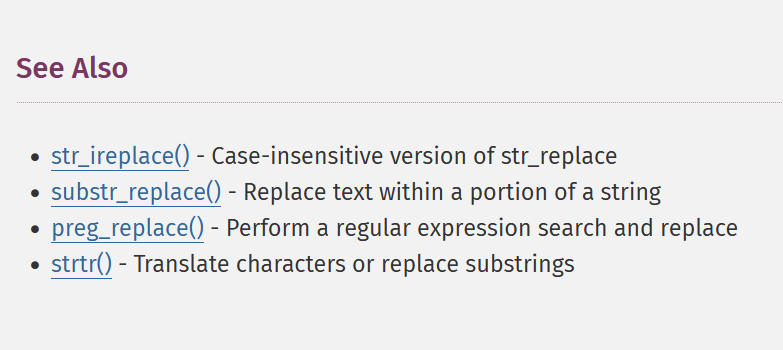
By carefully reading through these sections of the function's documentation, you can gain a thorough understanding of what the function does, how to use it correctly, and what to expect from its behavior.
Key takeaways
- Choose descriptive names that clearly indicate the purpose and functionality of the function. This helps improve code readability and understanding.
- Aim for functions with a single, well-defined responsibility. Functions should focus on performing a specific task, making them easier to understand, test, and maintain.
- Keep functions concise and focused. Avoid creating long functions that perform multiple tasks. Split complex logic into smaller, reusable functions to improve code readability and maintainability.
- Strive for functions that have minimal or no side effects outside their scope. Functions should ideally operate on their input parameters and return values, avoiding modifications to global variables or external states.
- Utilize PHP's type hinting feature to specify the expected parameter types and return types. This helps enforce type safety and provides better clarity for function inputs and outputs.