Variables
In this lesson, we will explore how variables are useful for storing data in a program.
What are variables?
A variable is like a container that holds a value that can be changed during the execution of a program.
Think of it like a box that can store different things. You give the box a name (the variable name), and then you can put a value inside it. The value can be changed later in the program, just like you can change what's inside the box.
Key Concept: Variables are not stored forever
The values of the variables are stored in memory for the duration of the script's execution. Once the script has finished executing, the memory is freed, and the values of the variables are lost unless they have been saved elsewhere.
In web applications, this means that each time a user makes a request to a PHP script, the variables are created, and their values are stored in memory only for the duration of that request. Once the request has been processed and the response has been sent back to the browser, the memory is freed, and the variables are lost.
To persist data between requests, it is necessary to store it in a database or other persistent storage mechanism. This is something we will discuss in a future chapter.
Creating variables
There are two core pieces of a variable.
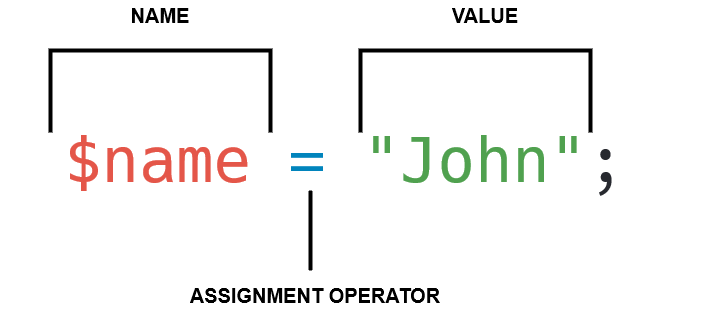
- The variable name, which should describe the type of data stored in the variable.
- The variable value, which is the value stored in the variable.
Let's dissect each piece of the variable.
Variable names
There are a few rules and conventions that you must follow for variable names.
- Variable names must start with a dollar sign (
$
). - Variable names must start with a letter or an underscore.
- After the first character, variable names can consist of letters, digits, and underscores.
- Variable names are case-sensitive (e.g.,
$name
and$Name
are different variables). - Variable names should not contain spaces.
- Variable names should be descriptive and meaningful.
- Variable names should not use reserved keywords as the name. For example, you should avoid naming a variable
$echo
.
Valid Variable Names
$name;
$age;
$_height;
$total_marks;
Invalid Variable Names
$12name; // starts with a number
$new-name; // contains a hyphen
$new name; // contains a space
$for; // a reserved keyword
No PHP tags?
You may have noticed it, but in the previous code snippets, there are no opening or closing PHP tags. For the sake of brevity, I'll be omitting the PHP tags from code snippets. You should always assume we're writing PHP inside PHP tags unless stated otherwise.
Naming Conventions
In PHP, there are several common naming conventions for variable names, including snake casing, pascal casing, and camel casing.
Camel casing
In camel casing, the first letter of each word is capitalized, except for the first word, which is written in lowercase letters. For example:
$firstName;
$lastName;
$customerAddress;
Pascal casing
In pascal casing, the first letter of each word is capitalized, and there are no underscores. For example:
$FirstName;
$LastName;
$CustomerAddress;
Snake casing
In snake casing, words are separated by underscores, and the entire name is written in lowercase letters. For example:
$first_name;
$last_name;
$customer_address;
It's important to note that these are just conventions, and you are free to choose the naming style that you prefer. The most important thing is to be consistent and choose a style that makes your code readable and easy to understand for yourself and other developers who may work with your code in the future.
Furthermore, if you plan on learning a PHP framework, each framework has different guidelines for naming conventions.
- Symfony encourages camelCase.
- WordPress encourages underscores and does not like camelCase.
- CodeIgniter also promotes snake casing.
Quickly mentioning PSR
In the PHP world, there's a widely adopted set of standard practices called PSR. It's something we'll be looking at in a future lesson, but if you're curious as to where to find more info, check out this link: https://www.php-fig.org/psr/
Best Practices
Believe it or not, developers struggle with variable naming. It can seem like a no-brainer at times, but it can easily cause confusion if you pick up bad habits.
When naming variables in PHP, it's important to follow good practices to make your code clear, readable, and maintainable. Here are some tips and tricks for naming variables:
- Be descriptive: Variable names should accurately reflect the purpose and contents of the variable. For example, instead of using
$x
, use a descriptive name like$customer_name
or$order_total
. - Use meaningful abbreviations: If you need to use abbreviations, make sure they are widely used and easily recognizable. For example, using
$cust_name
instead of$custn
is easier to understand. - Avoid using single letters: Using single letters as variable names, such as
$x
,$y
, or$z
, can be confusing and make it difficult to understand the variable's representation. - Be consistent: Choose a naming convention and stick to it. For example, if you choose snake casing, use it consistently throughout your code.
- Avoid using misleading or generic names: Using misleading or generic names, such as
$data
, can be confusing and make it difficult to understand what the variable represents.
Naming variables in PHP requires careful consideration and attention to detail to ensure your code is readable, maintainable, and easy to understand. By following these tips and tricks, you can ensure that your variables are named in a way that accurately reflects their purpose and contents.
Variables values
Variables can be assigned values. Assigning a value to a variable in PHP means storing a value in a variable for later use in the code. To assign a value to a variable in PHP, you use the assignment operator (=
). The syntax for assigning a value to a variable is:
$name = "John";
In the example above, $name
is the name of the variable, and "John"
is the value that you want to assign to the variable.
Updating variable values
It's important to note that once a value has been assigned to a variable, the value can be changed by reassigning a new value to the same variable. For example:
$name = "John Doe";
$name = "Jane Doe";
Updating a variable is the same process as creating a variable. Don't worry about PHP becoming confused. It'll know when you're creating a new variable or updating an existing variable.
Using a variable
After creating a variable, we can use the value stored in it by referencing it through its name. For example:
$name = "John";
echo $name;
In this example, we're echoing the $name
variable. Whenever the PHP interpreter comes across a variable, it'll access the value stored in the variable. The value stored in the variable is what will be outputted onto the page.
What are operators?
An operator in PHP is a symbol that performs a specific operation on one or more values (operands) and produces a new value. Think of an operator as a tool that helps you manipulate values in your code. Just like a hammer is a tool that helps you build something by hitting nails, operators in PHP help you manipulate values by performing operations on them.
The first operator we're introducing is the assignment operator. It's written with the =
character. The job of an assignment operator is to assign a value to a variable.
Each operator performs a specific operation and has specific rules for how it works. Understanding and using operators correctly is essential for writing effective and efficient PHP code.
Throughout this book, we'll continue to introduce new operators as we need them. For now, just knowing the assignment operator will suffice.
Reusing vs. creating new variables
You should avoid being a lazy programmer whenever possible. A common pitfall beginner developers fall into is repurposing an existing variable by constantly changing its value.
In general, it's considered good practice to create a new variable when it's necessary for clarity and readability of the code. Reusing a variable for multiple purposes can make the code less readable and harder to understand, especially if the variable is used for different purposes in different parts of the code.
For example, consider the following code:
$name = "John";
$name = "Amazon";
Here, it's clear that the variable $name
is being used for two different purposes: first, to store the name of a user and then to store the name of a business. In this case, it's better to create two separate variables, like this:
$username = "John";
$businessName = "Amazon";
This makes the code more readable and easier to understand, as the purpose of each variable is clear.
However, in some cases, reusing a variable can be acceptable if the variable's contents are no longer needed after a certain point in the code and if the code remains clear and readable.
In conclusion, it's a matter of balancing the trade-off between clarity and readability of the code, and the potential for unnecessary memory usage. When in doubt, it's better to create a new variable to ensure that the code is clear and easy to understand.
Exercises
Exercise #1
- Declare two variables:
admin
andname
. - Assign the value
"John"
toname
. - Copy the value from
name
toadmin
. - Show the value of admin using
echo
(must output “John”).
Exercise #2
- Create a variable with the name of our planet. How would you name such a variable?
- Create a variable to store the name of a current visitor to a website. How would you name that variable?
Key takeaways
- A variable is like a container that holds a value that can be changed during the execution of a program.
- Popular naming conventions for variable names are snake casing, camel casing, and pascal casing.
- Snake casing variable names are when words are separated with underscore characters.
- In pascal casing, the first letter of each word is capitalized, and there are no underscores.
- In snake casing, words are separated by underscores, and the entire name is written in lowercase letters.