Understanding HTTP for PHP Developers
HTTP stands for HyperText Transfer Protocol. It's like the rulebook for how information is passed around the web. But what does that mean?
Let's imagine the internet as a huge network of computers all connected to each other. These computers need a way to send and receive information such as text, images, videos, and so on. HTTP is the protocol or set of rules that computers use to do this.
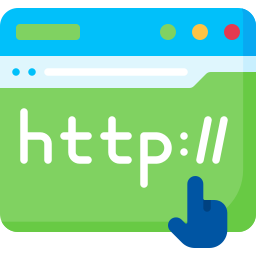
Here's a simple example. When you type a web address (like www.google.com) into your web browser and hit enter, what you're doing is sending an HTTP request to Google's server. This request says something like, "Hey Google, I would like to see the homepage."
Google's server receives your request, understands it because it also speaks HTTP, and sends back an HTTP response. This response includes the HTML, CSS, and JavaScript code that your browser needs to display Google's homepage to you.
So, in short, HTTP is the system that allows us to request information from a server (like a webpage) and have that information sent back to us. This is how all information is transferred on the web, and it's an essential part of web development, including PHP development.
HTTP Requests
The first part of HTTP communication is the HTTP request. In the simplest terms, an HTTP request is a message that your browser (the client) sends to a web server asking for a specific web page or resource.
When you type a URL into your browser's address bar and press enter, your browser prepares an HTTP request to send to the server. The URL tells your browser where to send the request.
Let's break down the anatomy of a URL. Suppose you type the following URL:
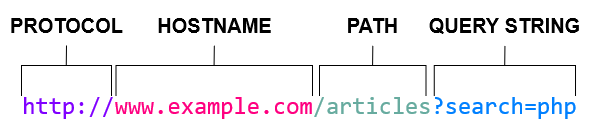
- Protocol: This is also known as the scheme. It defines the method of communication for the request. In the context of web addresses, the protocol is typically http or https (where the "s" stands for "secure").
- Hostname: This is the domain name (e.g., www.google.com) or IP address of the server that hosts the resource.
- Path: This is the specific location of the resource on the server. It's often structured similarly to a file path on your local computer (e.g.,
/directory/file.html
). - Query String: This is optional. It starts with a question mark (
?
) and includes parameters that may influence the response. It often takes the form ofkey=value
pairs separated by an ampersand (&
), like so:?key1=value1&key2=value2
.
You can think of it like this, an HTTP request is like a letter your browser sends to a server, and the URL is the address on the envelope telling the server what you're asking for.
Request Headers
The URL contains a lot of handy information, but it's not the only information sent over the network. Behind the scenes, browsers send something called HTTP request headers with additional information about the request.
HTTP request headers are a vital part of HTTP requests that provide additional information about the request. They work like the settings or properties of the request.
Imagine the HTTP request as a letter being sent in the mail. The contents of the envelope are like the body of the HTTP request - that's the main thing you're sending. But the envelope also has other information on it like the return address, postage stamp, etc. This is like the headers of an HTTP request.
You can view the request headers sent by opening the developer tools. Most browsers allow you to open the developer tools with a F12
keyboard shortcut.
What are developer tools in browsers?
Developer tools, often known as DevTools, are a set of software utilities built directly into most modern web browsers like Google Chrome, Firefox, Safari, and Edge. They help developers to inspect, test, and debug web pages and applications right in the browser.
There are quite a few tools available from the DevTools. The one we care about is the Network panel.
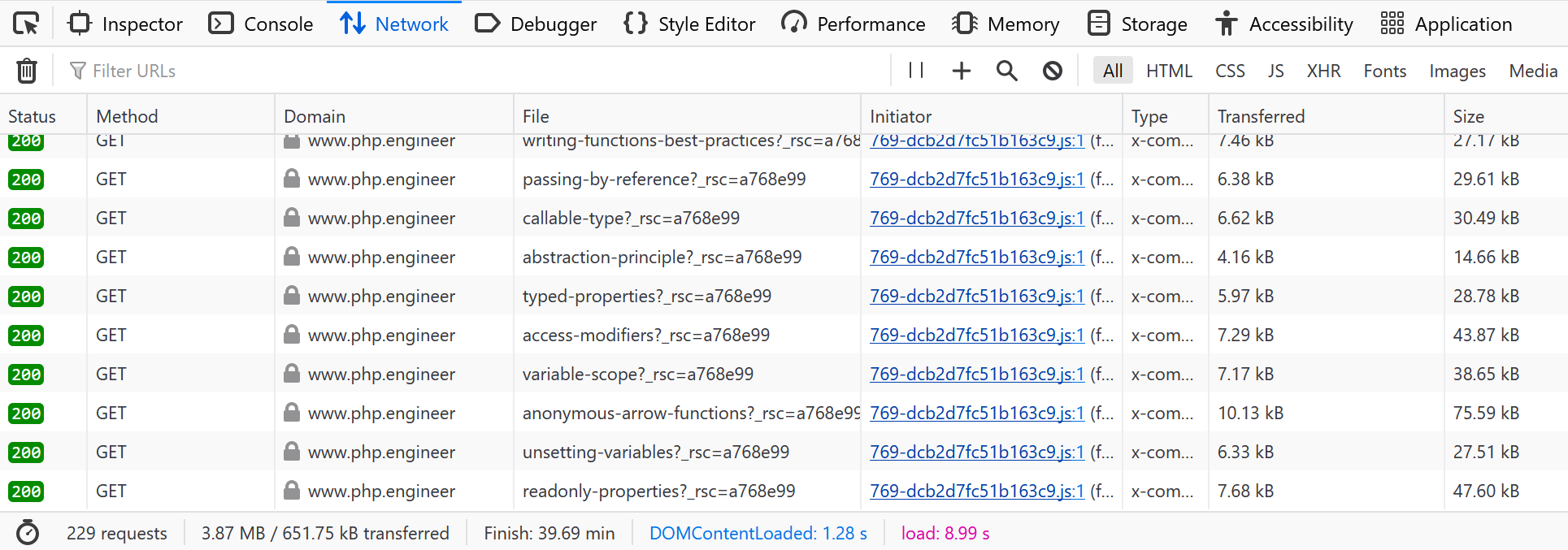
This tool shows all the network requests made by the page (like loading images, scripts, and stylesheets, and making AJAX calls). You can inspect the details of each request and response, including the headers, body, and timing information, by clicking on them.
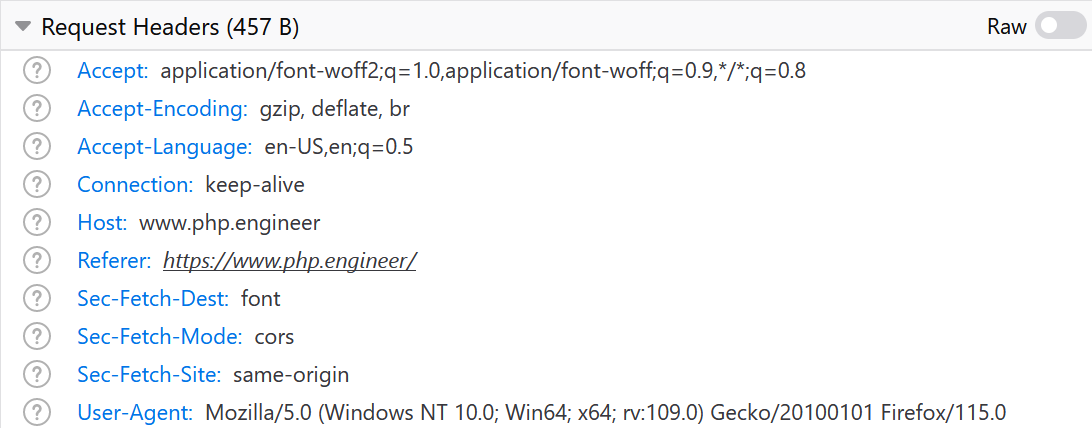
Depending on what browser you're using, there should be a section dedicated to request headers. As you can see, browsers are sending various pieces of information.
HTTP Response
An HTTP response is the message that a web server sends back to your browser after it has processed your HTTP request.
Imagine you're sending a letter (the HTTP request) to a friend asking for a specific picture. Your friend finds the picture and mails it back to you. That return letter with the picture is like the HTTP response. It includes both the picture you asked for (the body of the response) and some extra info like a note telling you they found the picture (the status), how big the picture is (content length), what format it's in (content type), and so on (other headers).
Web servers perform a few steps when a request for a PHP file is initiated.
- Based on the requested URL, the server identifies which PHP file it needs to execute. This mapping is typically based on the server's file system, but it can also be influenced by the server's configuration or URL rewriting rules.
- The server passes the PHP file to the PHP interpreter. The interpreter executes the PHP code, which might involve reading from or writing to a database, manipulating data, or performing other server-side operations.
- The PHP script generates some output, which could be HTML, JSON, XML, or another data format. The server takes this output, adds some HTTP headers, and constructs an HTTP response.
- The server sends the HTTP response back to the client, AKA the browser .
HTTP Response Headers
Similar to HTTP requests, you can view HTTP responses with the developer tools. Once again, where this information can be found varies on the browser you're using:
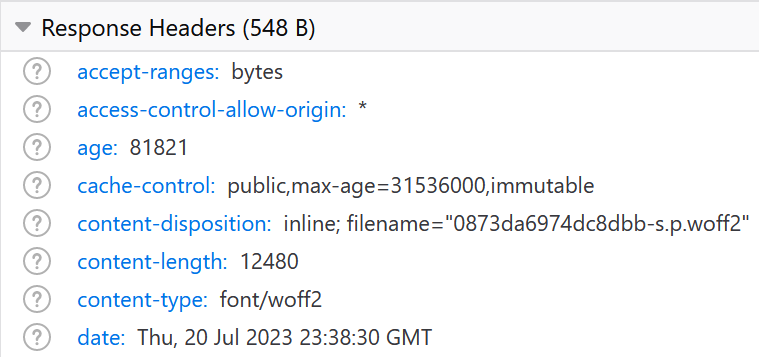
HTTP Status Codes
Another piece of information sent with both requests and responses is HTTP status codes. HTTP status codes are three-digit numbers that web servers use to communicate the status of an HTTP response. They're part of the first line of the response, known as the status line.
There are five classes of HTTP status codes, grouped by the first digit:
- 1xx (Informational): The request was received and understood. It must be continued to get the final response. Example: 100 (Continue)
- 2xx (Successful): The request was successfully received, understood, and accepted. Example: 200 (OK), 201 (Created), 204 (No Content)
- 3xx (Redirection): Further action needs to be taken in order to complete the request. Often, these status codes are used for URL redirection. Example: 301 (Moved Permanently), 302 (Found), 304 (Not Modified)
- 4xx (Client Error): The request contains bad syntax or cannot be fulfilled. These indicate that the client has made a mistake. Example: 400 (Bad Request), 403 (Forbidden), 404 (Not Found)
- 5xx (Server Error): The server failed to fulfill an apparently valid request. These indicate problems on the server side. Example: 500 (Internal Server Error), 502 (Bad Gateway), 503 (Service Unavailable)
The status code is always accompanied by a reason phrase, a short, human-readable explanation of the status, like "Not Found" or "Internal Server Error". This can help developers diagnose problems, but it's mainly meant to be read by people, not parsed by software.
HTTP status codes are defined in the HTTP specification (RFC 7231 and others), and they're an important part of the HTTP protocol, which powers the World Wide Web. You can find a complete list of status codes here: https://developer.mozilla.org/en-US/docs/Web/HTTP/Status
Don't stress it!
Don't worry too much about memorizing every single status code there is to offer. Most developers only memorize a few off the top of their head, like 200
or 404
. You should bookmark the link I gave above as a reference.
Key Takeaways
- HTTP is request-response based. Clients (like web browsers) send requests, and servers send responses.
- Both HTTP requests and responses have a start line, headers, and a body. The start line and headers are always text, but the body can be any type of data.
- In HTTP, URLs (Uniform Resource Locators) are used to identify resources and provide information on how to fetch them. They include the protocol (http or https), host, port, path, and query string.
- HTTP status codes convey response status.
- These three-digit codes indicate whether a request was successful and, if not, what kind of error occurred.
- They're divided into five classes: 1xx (informational), 2xx (success), 3xx (redirection), 4xx (client errors), and 5xx (server errors).
- Both requests and responses can include headers, which provide additional details about the request or response, such as the content type, content length, caching directives, and much more.
- Remember that understanding HTTP is crucial for PHP developers since PHP is commonly used to build web applications that work over HTTP. Whether you're handling form data, setting cookies, redirecting users, or troubleshooting errors, a solid knowledge of HTTP will help you create better, more robust applications.