String Mutation Functions
Out of the box, PHP offers dozens of functions for manipulating strings. Almost anything you can imagine can be done with PHP's built-in functions. For a complete list of string functions, you can find them here:
https://www.php.net/manual/en/ref.strings.php
While we can't cover every function possible, we'll focus on the most common functions for mutating strings.
What is mutation?
Mutation is a common word you'll come across in the programming world. Mutation in programming refers to the process of changing the value of a variable after it has been created. For instance, if you have a variable that holds the value 5
, and later in your program, you change that variable to hold the value 10
, you've mutated the variable.
Basic String Formatting
If you're working with sentences or titles of text, PHP offers some basic functions to help you with basic formatting. We can use the strtolower()
, strtoupper()
, ucfirst()
, and ucwords()
functions are used for string manipulation. These functions are useful when you want to format text in a specific way, such as making everything lowercase for consistency or capitalizing the first letter of each word for a title.
Each of these functions accepts a string and returns a string. Here's what each of them does:
Function | Description |
---|---|
strtolower($string): string | Converts and returns a string to all lowercase letters. |
strtoupper($string): string | Converts and returns a string to all uppercase letters. |
ucfirst($string): string | Converts and returns the first character of a string to uppercase. |
ucwords($string): string | Converts and returns the first character of each word in a string to uppercase. |
Here's an example of using each function:
$text = "welcome to php programming!";
// convert the string to all lowercase
$lowercase = strtolower($text);
echo "<p>Lowercase: $lowercase</p>";
// convert the string to all uppercase
$uppercase = strtoupper($text);
echo "<p>Uppercase: $uppercase</p>";
// convert the first character of the string to uppercase
$firstUpper = ucfirst($text);
echo "<p>First character uppercase: $firstUpper</p>";
// convert the first character of each word in the string to uppercase
$wordsUpper = ucwords($text);
echo "<p>First character of each word uppercase: $wordsUpper</p>";
In this script, we start by defining a string in $text
, then we apply each of the string transformation functions to $text
, store the results in new variables, and echo
them out wrapped in HTML paragraph tags for readability.
You will receive the following output:
welcome to php programming!
WELCOME TO PHP PROGRAMMING!
Welcome to php programming!
Welcome To Php Programming!
Substrings
A substring is a contiguous sequence of characters within a string. In other words, it's a smaller part or section of a main string.
For example, in the string "Hello, World!", "Hello" and ", World" are substrings.
The concept of a substring is quite fundamental in text processing tasks such as searching for patterns within a text, replacing parts of a string, and so on. In PHP, you can use functions like substr()
to extract a substring from a string.
The syntax for this function is the following:
substr ( string $string , int $offset , int|null $length = null ) : string
$string
: (Required) The string to process.$offset
: (Required) Specifies where to start in the string.$length
: (Optional) Specifies the length of the returned string. If this parameter is omitted, thesubstr()
function will return all characters to the end of the string.
Basic Example
The following example uses the substr()
function to extract the first four characters from a string.
The first argument is a string 'EXAMPLE'
. The second argument is the offset, which is the starting position. In this example, we're starting at the beginning of the string by using 0
. As a reminder, strings always begin at 0
, not 1
. Lastly, we're providing a length, which is 4
.
The result of this example will be 'EXAM'
.
echo substr('EXAMPLE', 3); // EXAM
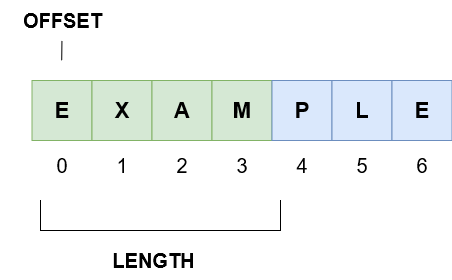
Default Length
The third argument of the substr()
function is optional. If we decide not to provide a value, PHP automatically assumes we want everything from the offset to the end of the string.
In this example, we're setting the offset to 2
.
The result will be 'AMPLE'
.
echo substr('EXAMPLE', 2); // AMPLE
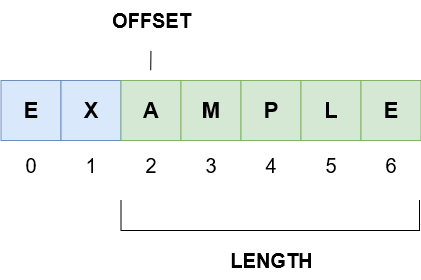
Negative Offset
The argument $offset
can take a negative value. When $offset
is negative, the substr()
function generates a substring starting from the offset character, counting from the offset to the end of the string. The last character in the string has an index of -1
In this example, the offset is -4
. Therefore, the starting character is M
.
This example returns 'MPLE'
.
echo substr('EXAMPLE', -4); // MPLE
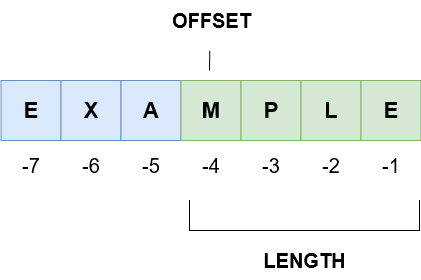
Negative Length
The $length
parameter can be given a negative value. By passing in a negative value, the $length
argument is treated as how many characters should be omitted from the results from the end of the string.
This example returns 'MP'
.
echo substr('EXAMPLE', -4, -2); // MP
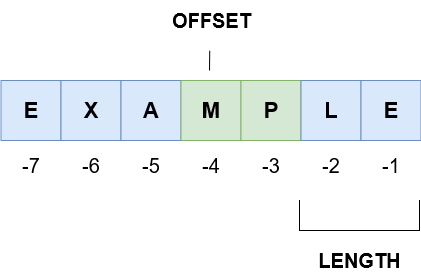
String Replacement
The str_replace()
function in PHP is used to replace some characters with some other characters in a string.
The syntax for this function is as follows:
str_replace (
array|string $search ,
array|string $replace ,
string|array $subject ,
int &$count = null
) : string|array
Here's what each parameter is for:
$search
: (Required) It specifies the value to find. It can be a string or an array of strings.$replace
: (Required) It specifies the value to replace the values in the find parameter. It can be a string or an array of strings.$subject
: (Required) It specifies the string to be searched. It can be a single string or an array of strings.$count
: (Optional) It's a variable that, after the function call, will be filled with the number of replacements done.
Here are a few things to keep in mind when using this function:
- When both
$search
and$replace
are arrays,str_replace()
systematically replaces each value in$search
(from left to right) with the corresponding value in$replace
. - If
$replace
has fewer items than$search
,str_replace()
will use an empty string for the extra replacement values. - When
$search
is an array and$replace
is a string,str_replace()
will replace every$search
element with the$replace
string. str_replace()
does not modify the original input string ($subject
). Instead, it generates a new string where the$search
items have been replaced with$replace
items.
Basic Example
Suppose we have a sentence where we want to replace the word 'cat'
with 'dog'
. Here's how you can do it:
As you can see, both instances of the word 'cat'
in the original string have been replaced by 'dog'
.
$originalString = "The cat is cute. I like the cat.";
$modifiedString = str_replace("cat", "dog", $originalString);
echo $modifiedString;
The dog is cute. I like the dog.
Using the count argument
The str_replace()
function also accepts a fourth parameter that will hold the count of replaced values.
In this script, we're initializing a $count
variable to zero before invoking str_replace()
. We then pass $count
as the fourth argument. After the function call, $count
will hold the number of replacements made.
When you run this script, you will see that the count of replacements (2
) is displayed.
$originalString = "The cat is cute. I like the cat.";
$count = 0;
$modifiedString = str_replace("cat", "dog", $originalString, $count);
echo "The 'cat' word was replaced $count times.";
The 'cat' word was replaced 2 times.
Multiple Replacements
We can replace multiple strings by using an array for the $search
and $replace
arguments. The string "cat"
will always be replaced with the string "dog"
. The string "garden"
will always be replaced with the string "park"
. PHP goes from left-to-right
As you can see, PHP replaces the items with their correct items from the array.
$originalString = "The cat is in the garden. I love the cat and the garden.";
$searchArray = ["cat", "garden"];
$replaceArray = ["dog", "park"];
$modifiedString = str_replace($searchArray, $replaceArray, $originalString);
echo $modifiedString;
The dog is in the park. I love the dog and the park.
Using the str_ireplace function
The str_ireplace()
function in PHP is very similar to the str_replace()
function, with one key difference: str_ireplace()
is case-insensitive. This means it will replace a string regardless of whether it's in lowercase, uppercase, or any combination thereof. It has the exact same arguments as the str_replace()
function.
As you can see, even though our search array contained uppercase "CAT"
and "GARDEN"
, and the original string contained "Cat"
, "Garden"
(with different cases), str_ireplace()
successfully replaced them. This function is very useful when you want to ensure that all instances of a word or phrase are replaced, regardless of their case.
$originalString = "The Cat is in the garden. I love the Cat and the Garden.";
$searchArray = array("CAT", "GARDEN");
$replaceArray = array("dog", "park");
$modifiedString = str_ireplace($searchArray, $replaceArray, $originalString);
echo $modifiedString;
The dog is in the park. I love the dog and the park.
Trimming Strings
PHP offers three functions for trimming strings. They're trim()
, ltrim()
, and rtrim()
. Let's start our discussion with the trim()
function.
The trim()
function in PHP is used to remove white space and other predefined characters from both sides of a string. This could be leading spaces, trailing spaces, or other types of characters.
Here is the syntax of the trim()
function:
trim(string $string, string $characters = " \n\r\t\v\x00"): string
$string
: The string that you want to trim.$characters
(optional): You can optionally specify the characters that you want to be stripped.
By default, the trim()
function removes the following characters: " \n\r\t\v\0"
Here's what each character means:
Character | Description |
---|---|
" " | A space |
"\t" | A tab |
"\n" | A new line |
"\r" | A return |
"\0" | A NUL-byte |
"\v" | A vertical tab |
This function returns the original string with the characters stripped listed in the second argument.
Removing Excess Whitespace
In this example, the trim()
function removed the extra spaces from the start and end of the string, making it cleaner and easier to work with. This is particularly useful when handling user input, as users often accidentally add extra spaces.
$myString = " Hello, world! ";
$trimmedString = trim($myString);
var_dump($trimmedString);
string(13) "Hello, world!"
Removing Custom Characters
The $_SERVER
superglobal array contains information about the server and the HTTP request, among other things. The REQUEST_URI
element of this array contains the URI (Uniform Resource Identifier) of the request.
Let's say you want to remove slashes from the beginning and end of the REQUEST_URI
. Here's how you can do it:
In this example, $_SERVER['REQUEST_URI']
might give you something like "/mywebsite/homepage/"
. The trim()
function is then used to remove the leading and trailing slashes, so $trimmed_uri would contain "mywebsite/homepage"
.
It's important to note that this will only remove slashes at the beginning and end of the string. If there are any slashes inside the string, they won't be removed.
$uri = $_SERVER['REQUEST_URI'];
$trimmed_uri = trim($uri, "/");
echo $trimmed_uri;
ltrim and rtrim
There are two other functions for trimming characters called ltrim()
and rtrim()
. ltrim()
and rtrim()
are PHP functions that are similar to trim()
, but they each operate on only one end of the string. Here's what they do:
ltrim(string $string, string $characters): string
: This function removes whitespace or other specified characters from the beginning (left side) of a string.rtrim(string $string, string $characters): string
: This function removes whitespace or other specified characters from the end (right side) of a string.
Here's an example to illustrate this:
$text = ' Hello, world! ';
var_dump(ltrim($text)); // Output: string(14) "Hello, world! "
var_dump(rtrim($text)); // Output: string(14) " Hello, world!"
var_dump(trim($text)); // Output: string(13) "Hello, world!"
In this example, ltrim()
removes the space at the beginning, rtrim()
removes the space at the end, and trim()
removes both.
Key Takeaways
strtolower()
andstrtoupper()
: These functions convert a string to all lowercase and all uppercase characters, respectively.ucfirst()
anducwords()
: These functions capitalize the first character of a string and the first character of each word in a string, respectively.substr()
: This function returns a portion of a string starting at a specified position. It can accept negative offsets to start counting from the end of the string.str_replace()
: This function replaces certain portions of a string (specified by the search parameter) with a replacement string.str_ireplace()
: This function works similarly tostr_replace()
, but it's case-insensitive.trim()
: This function removes whitespace (or other specified characters) from the beginning and end of a string.ltrim()
andrtrim()
: These functions work similarly to trim(), but ltrim() only removes characters from the beginning of a string, and rtrim() only removes characters from the end.- Remember that all these functions return new strings and do not change the original strings. If you want to keep the result of these functions, you need to store it in a new variable or overwrite the old one.