Cookies
Servers communicate with browsers using the HTTP protocol. When a server wants a browser to store data, it includes this data in the HTTP response it sends to the browser. The data is included in a specific part of the response known as the HTTP headers.
A cookie is one of the methods used to store data on the client's side (the user's browser). It's a small piece of text that a server sends to a user's browser in the HTTP headers. Each cookie includes a name and a value, as well as optional attributes like the expiration date and the domain and path where the cookie is valid.
Cookie Overview
When a browser receives a cookie from a server, it stores the cookie on the user's computer or device. Then, every time the browser sends a request to the same server, it includes the cookie in the HTTP headers. This way, the server can remember information about the user's previous activity.
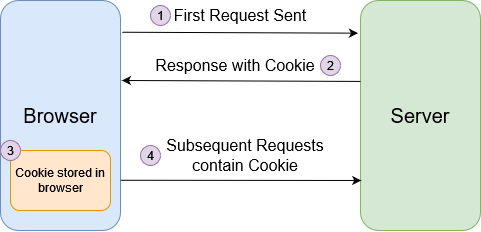
Let's break it down into steps.
- For the first request, you open your browser and navigate to a website, say,
www.example.com
. This is the first request you are sending to the website's server. At this point, there are no cookies related to this site in your browser because you have never visited it before. - The server at
www.example.com
receives your request and sends back a response. In this response, the server can include an HTTP header to ask your browser to store some data. For instance, the server might send back a cookie namedfirst_visit
with a value of the current date and time. This tells your browser, "Please save this piece of data and send it back to me whenever you make future requests." - Your browser receives the server's response, along with the
first_visit
cookie. The browser then stores this cookie somewhere on your computer. - The next time you visit
www.example.com
, your browser sends another request to the server. This time, because it has a cookie from this server, it includes the cookie in its request. It's the browser's way of saying, "Hey, server, you asked me to remember this first_visit cookie last time, here it is!" - When the server receives the request, it also gets the
first_visit
cookie. The server can read the cookie's value and know when you first visited the site. - The server can update the cookie or set new ones in subsequent responses, and your browser will continue to return these cookies with each request. This way, the server can remember information across multiple requests.
So, in simple terms, the server asks the browser to remember a small piece of data (a cookie), and the browser sends that data back to the server whenever it makes a request to the same server. This process allows the server to remember things about your interactions with the website, despite the inherently stateless nature of HTTP.
Do not store sensitive data
Cookies are always stored as plain text. They're constantly sent between clients and servers. In addition, the JavaScript programming language has access to cookies stored on the browser. It's inadvisable to store sensitive information in a cookie, such as emails or credit card information.
Creating a Cookie
Cookies in PHP can be created using the setcookie()
function. The setcookie()
function sends an HTTP response header containing the cookie information to the client's (user's) browser.
Here's a basic example of creating a cookie in PHP:
setcookie("test_cookie", "test_value");
In this example:
"test_cookie"
is the name of the cookie."test_value"
is the value of the cookie.
This code would tell the user's browser to store a cookie named test_cookie
with a value of "test_value"
.
Once a cookie is set, it will be included in every subsequent HTTP request to the server until it expires or is deleted.
Overwriting an Existing Cookie
Overwriting an existing cookie in PHP is done in the same way as setting a new one -- you use the setcookie()
function. When you set a cookie with the same name as an existing one, the new value you specify will overwrite the old value.
// Setting a cookie
setcookie("test_cookie", "test_value");
// Overwriting the same cookie
setcookie("test_cookie", "new_value");
In this example, we're overwriting the cookie we created previously. setcookie()
call, the "test_cookie"
now has a new value of "new_value"
.
When a server tells a browser to set a cookie that it already has, the browser will replace the old cookie with the new one. It uses the cookie's name to identify which cookie to update. This update involves changing the cookie's value.
Accessing Cookies
You can access cookies using the $_COOKIE
superglobal array. This array contains all the cookies that were sent by the client's browser in the HTTP request.
if (isset($_COOKIE["test_cookie"])) {
echo "Cookie 'test_cookie' value is: " . $_COOKIE["test_cookie"];
}
This script checks if the "test_cookie"
cookie exists, and if it does, it prints its value.
Using filter_input()
PHP also provides the filter_input()
function, which can be used to access and filter input data, including cookies. This function can be useful for sanitizing and validating the cookie data before using it.
$cookie_value = filter_input(INPUT_COOKIE, 'test_cookie', FILTER_SANITIZE_STRING);
if ($cookie_value !== null) {
echo "Cookie 'test_cookie' value is: " . $cookie_value;
}
In this script, filter_input(INPUT_COOKIE, 'test_cookie', FILTER_SANITIZE_STRING)
retrieves the value of the "test_cookie"
cookie and applies a filter that removes tags and encodes special characters. This can help protect against certain types of attacks, such as cross-site scripting (XSS). If the cookie is not set or its value is empty, filter_input()
will return null
.
Configuring Cookies
The setcookie()
function in PHP can accept multiple parameters that allow you to control various properties of the cookie.
Parameter | Description |
---|---|
$name | The name of the cookie. This is stored on the user's computer and is sent with every request. |
$value | The value of the cookie. This data is stored on the user's computer and is sent with every request. |
$expires | The time when the cookie expires. If set to 0 , or omitted, the cookie will expire at the end of the session. |
$path | The path on the server where the cookie will be available. If set to '/' , the cookie will be available within the entire domain. |
$domain | The domain that the cookie is available to. Setting this to a subdomain (e.g., www.example.com ) will make the cookie only available to that subdomain. |
$secure | Indicates whether the cookie should only be sent over secure (https) connections. |
$httponly | If set to TRUE , the cookie will be accessible only through the HTTP protocol. This helps to prevent attacks such as cross-site scripting (XSS). |
Here's an example of using these parameters to set a more secure cookie:
setcookie(
"secure_cookie", // name
"secure_value", // value
time() + 3600, // expires
"/", // path
"www.example.com", // domain
true, // secure
true, // httponly
);
In this example, the secure
parameter is set to true
, which means the cookie will only be sent over secure (HTTPS) connections. The httponly
parameter is also set to
true, which means the cookie can't be accessed via JavaScript. This can help protect against certain types of attacks, such as cross-site scripting (XSS).
Expiration Date
Let's talk more about the expiration. The expiration of a cookie determines how long it will be stored on the user's browser.
When you set a cookie, you can specify its expiration time as an optional parameter. The expiration time is specified as a Unix timestamp, which represents the number of seconds since the Unix Epoch (January 1, 1970, at 00:00:00 GMT).
Configuring the Expiration Time
In PHP, you can use the time()
function, which returns the current Unix timestamp, and add the number of seconds you want the cookie to last. For example, to set a cookie that expires in one hour, you could do:
// 3600 seconds = 1 hour
setcookie("my_cookie", "my_value", time() + 3600);
Omitting the Expiration Time
If you don't set an expiration time for a cookie, it becomes a session cookie. Session cookies are removed when the user closes the browser. So if you want a cookie to last for the duration of the user's browsing session, you can set the cookie without an expiration time:
setcookie("my_session_cookie", "my_value");
Negative Expiration Time
If you were to set a negative expiration time, the cookie would expire immediately. This can be useful if you want to delete a cookie:
In this case, by setting the expiration time to an hour in the past, you're telling the browser that the cookie has already expired, and the browser will delete the cookie.
// set the expiration time to one hour ago
setcookie("my_cookie", "", time() - 3600);
Viewing Cookies in the Browser
Naturally, you may want to verify a cookie was created in the browser by using the developer tools. Every browser has a suite of tools to help developers debug their site. A common feature is the ability to view cookies.
You can view cookies using the developer tools in most modern web browsers. The steps may vary slightly depending on the browser you are using. Here's how you can do it in Google Chrome:
- Open the Developer Tools: You can do this by right-clicking anywhere on a webpage and selecting
Inspect
from the context menu, or by pressingCtrl+Shift+I
(orCmd+Option+I
on a Mac) on your keyboard.
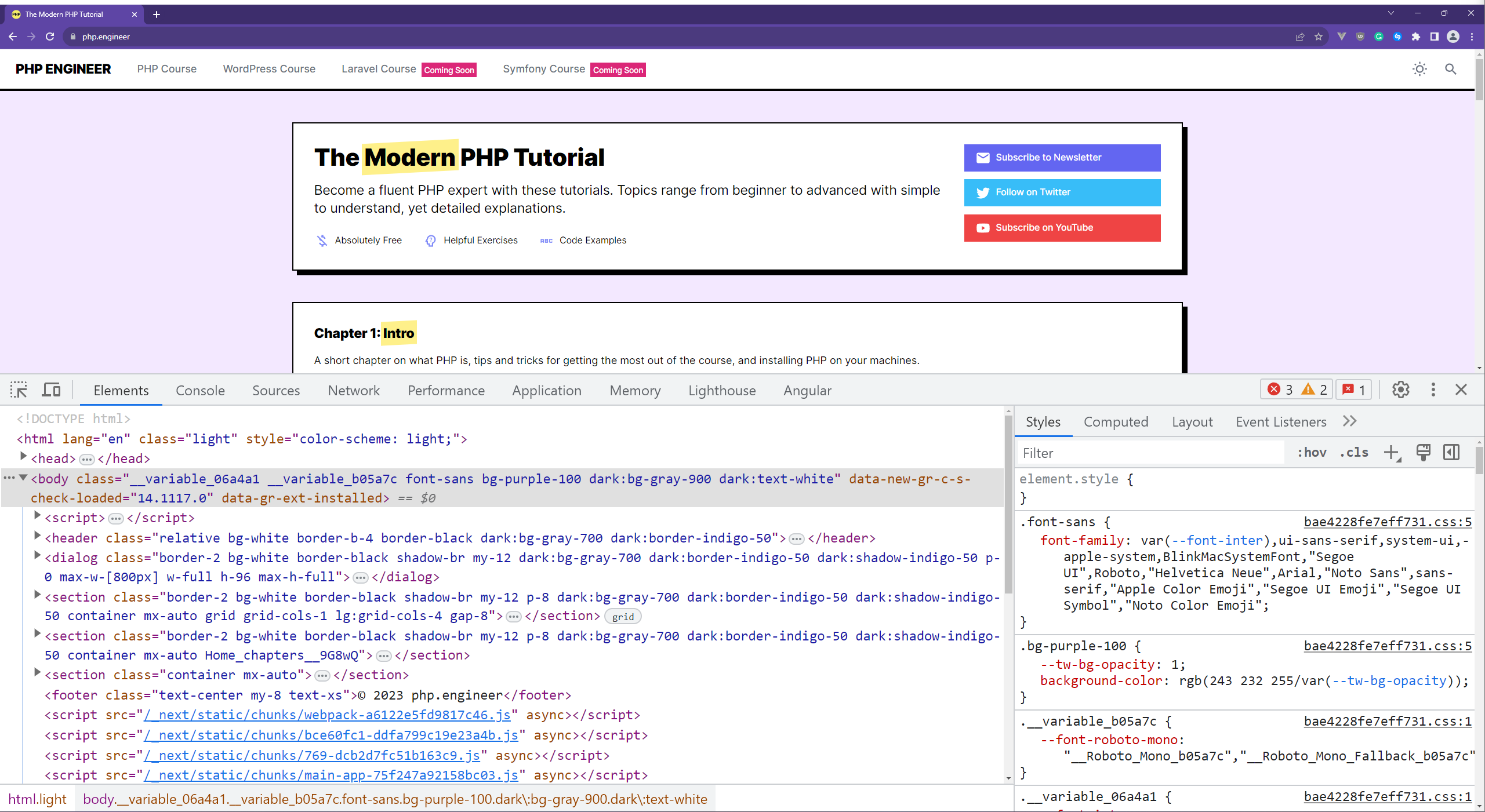
- In the Developer Tools panel, look for the
Application
tab and click it. If you don't see it, it might be hidden under the>>
button due to the small window size.
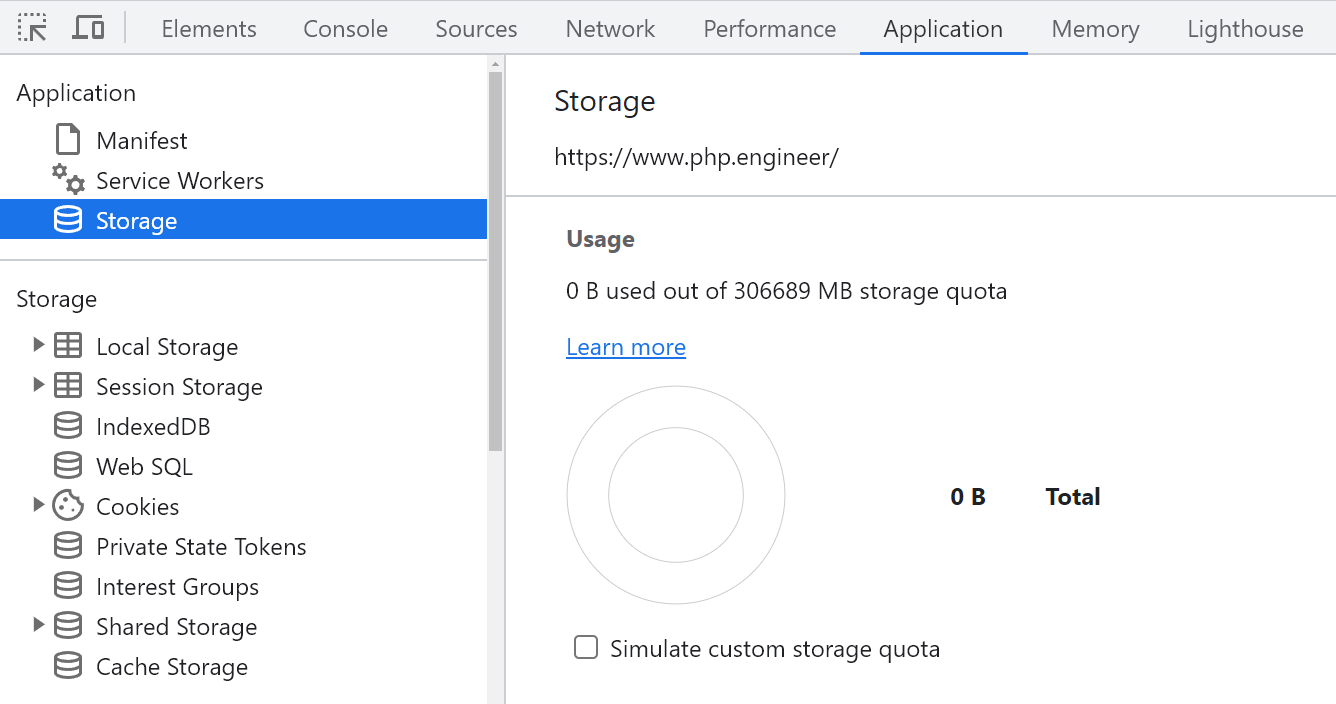
- Under the
Application
tab, there's a sidebar on the left with a section calledStorage
. Expand theStorage
section if it's not already expanded. - Click on
Cookies
in theStorage
section. This will expand it, and you'll see the domains for which cookies are currently stored. - Click on the domain that you're interested in. This will show all the cookies for that domain on the right side.
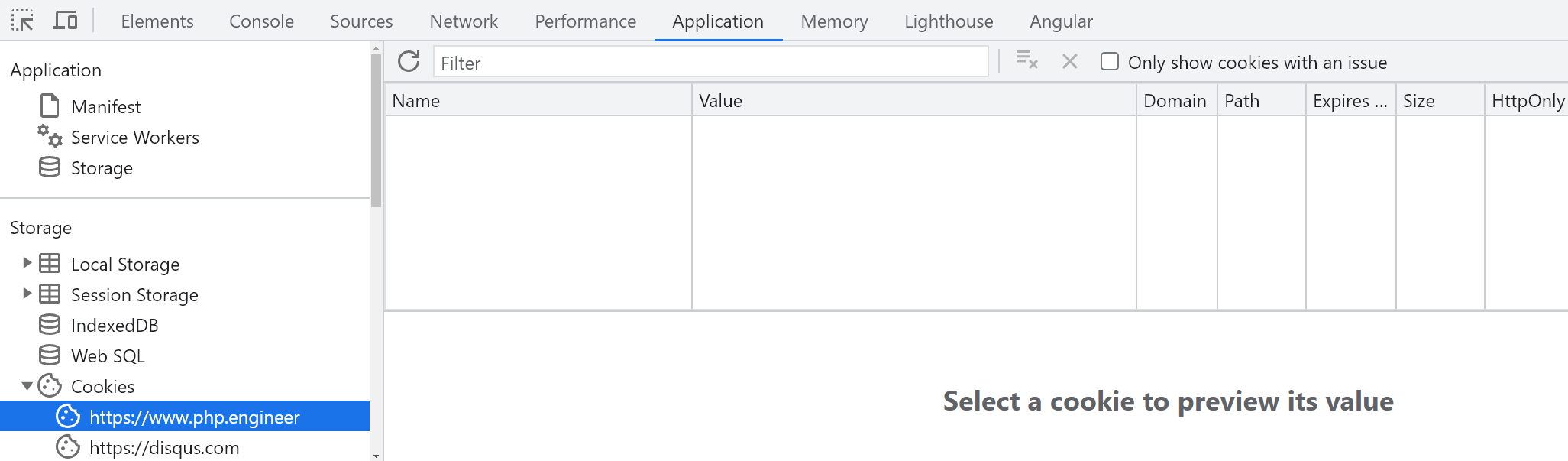
In this cookies
panel, you can see the Name
, Value
, Domain
, Path
, and Expiration
date of each cookie. You can also modify or delete cookies and see changes in real time if your website sets or updates cookies.
For other browsers like Firefox or Safari, the process is very similar, but the exact names and layout of the tabs in the developer tools might be slightly different.
Key Takeaways
- You can set a cookie in PHP using the
setcookie()
function. This function must be called before any output is sent to the browser. - The
setcookie()
function takes several parameters, including the cookie's name, value, expiration time, path, domain, and flags for secure and HTTP only. - Cookies sent by the client in the HTTP request can be accessed in PHP via the
$_COOKIE
superglobal array. - To update a cookie, you simply set a new cookie with the same name. The new cookie will overwrite the old one.
- You can delete a cookie by setting its expiration time to a timestamp in the past.
- If you don't set an expiration time for a cookie, it becomes a session cookie and will be removed when the user closes their browser.
- Cookies can be made more secure by setting them to be only sent over secure connections (HTTPS) and by making them accessible only through the HTTP protocol, which can help prevent attacks such as cross-site scripting (XSS).
- You can view the cookies set by a site in your browser's developer tools.