Concept: Validation
After receiving data from a request, it's essential to begin validating the data to ensure that it adheres to the expected formats, types, and constraints. Validation in PHP refers to the process of examining the data to check whether it meets specific criteria, such as a certain length, type, pattern, or range.
Why is it important?
Here are the primary reasons for performing validation:
- Data Integrity: By enforcing specific rules or formats, validation ensures that the data being processed aligns with the expected structure, preserving the integrity of the database or application.
- User Experience: Validation provides immediate feedback on the correctness of the data entered, guiding the user to correct mistakes. It enhances usability by catching errors early in the process.
- Avoiding Errors: Processing invalid data can lead to unexpected errors or incorrect results within an application. Validation helps prevent these issues by ensuring the data is appropriate before processing.
By ensuring that only valid and appropriate data is processed, validation acts as a vital safeguard in PHP applications, enhancing security, reliability, and overall functionality.
Taking Action
After validating data in a request, the next step is to decide whether to proceed with processing the request or throw an error. Here's how that process generally unfolds:
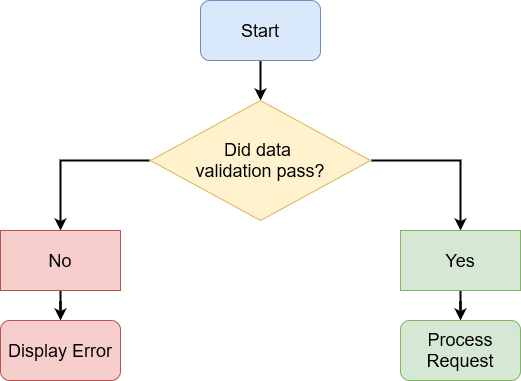
Firstly, you would assess the results of the validation process to determine if the data meets the required criteria or constraints.
If the data is valid, continue with the intended action, such as saving the data to a database, processing a form, or initiating a specific operation.
If the data is found to be invalid, generate an error message or response that informs the user (or calling system) of the specific problem with the data. This could involve redirecting to an error page, displaying an error message within the browser, or returning an error code and message if it's an API endpoint. It's always important to ensure that the error handling is user-friendly, providing clear and concise information about what went wrong and possibly how to correct it.
Depending on the severity and nature of the validation failure, you might also log the incident for review and monitoring. This can aid in debugging, compliance, and identifying potential malicious activities.
Displaying an Error Message
Let's use the example we had in the previous lesson. A valid request must have a query parameter called flavor
. If it doesn't, you can redirect a user to a different page, which would look something like this.
if (!isset($_GET['flavor'])) {
header("Location: flavor-not-found.php");
exit;
}
$flavor = $_GET['flavor'];
echo "My favorite ice cream flavor is {$flavor}.";
In this example, we're using the isset()
function to check if the $_GET['flavor']
variable is set. If it isn't, we're redirecting the user with the header()
function and setting the Location
header to a page called not-found.php
. Lastly, we're adding the exit
statement to stop the rest of the script from running. Otherwise, we're echoing the flavor.
Here's what the not-found.php
file could look like.
<h1>Please provide your favorite flavor</h1>
If we hadn't checked the $_GET['flavor']
variable, we would receive an error for trying to grab it.
It's important to display the error to the user. We shouldn't expect them to know how to fix the problem, such as modifying the URL to include a query parameter.
Key Takeaways
- Clear validation rules and error messages guide users to provide correct information, improving usability.
- Validating data before processing helps avoid unexpected errors or incorrect results within the application.