Caching
Caching in PHP is a technique used to store and reuse previously computed or retrieved data. Instead of recalculating or fetching the same information repeatedly, the system saves a copy of it, typically in a location that allows for faster access.
Caching is an essential performance optimization tool in PHP development, offering a balance between speed and resource efficiency. It's like keeping a snapshot of frequently used data handy so that the system doesn't have to recreate it every time.
Benefits
There are many benefits to adding caching to your site, such as:
- Caching significantly speeds up operations by reducing the need to recompute or fetch data.
- By reusing data, caching minimizes the load on databases or other data sources, improving overall system performance.
- Caching reduces the amount of data transferred between various parts of a system, saving bandwidth and other resources.
- Faster response times contribute to a better user experience, making applications more responsive and pleasant to use.
Overall, caching is invaluable for developers.
Types of Caching
Caching can be performed on the client side or server side. Utilizing both client-side and server-side caching in a balanced manner can lead to significant improvements in the speed, responsiveness, and resource utilization of a web application.
Client Side Caching
Client-side caching involves storing data on the user's device (e.g., browser cache). When a user visits a webpage, some resources like images, CSS, or JavaScript files may be stored locally. This means that the next time the user visits the same page, the browser can load those resources from the local cache rather than downloading them again from the server.
Types of Client-Side Caching:
- Browser Cache: Stores static resources like images, CSS, and JavaScript files.
- LocalStorage and SessionStorage: Stores data in the user's browser for more persistent or session-based storage.
Server-Side Caching
Server-side caching is done on the web server and can include several different techniques to store various types of data or content.
Types of Server-Side Caching:
- Object Caching: Stores data objects, like the results of database queries.
- Full Page Caching: Stores the entire HTML of a page to serve it quickly without regenerating it.
- Opcode Caching: Like OPcache in PHP, it stores the compiled version of the PHP scripts.
- CDN Caching: Content Delivery Networks (CDNs) cache content closer to users to reduce latency.
Let's explore OPCache, as it's one of the first types of caching you can perform with PHP.
What is OPCache?
Under the hood, PHP has a feature called OPCache. OPcache is a special caching system used in PHP to improve the performance of your web applications. Here's a beginner-friendly summary:
When a PHP script runs, it is first translated (or "compiled") into a language that the computer can understand. This compilation process takes time and resources.
OPcache helps by storing the compiled version of the script, so the next time the same script is requested, it doesn't have to be compiled again. It's like saving a snapshot of a finished puzzle, so you don't have to put the pieces together every time.
Here's a breakdown of the steps taken by PHP when OPCaching is enabled.
First Request
- When a PHP script is requested for the first time, the PHP interpreter compiles the script into opcode (intermediate code that the server can execute).
- The compiled opcode is then stored (cached) in OPcache. This cache resides in the server's memory, making it quick to access.
- The compiled opcode is executed to fulfill the request, and the output (like HTML) is sent to the client's browser.
- The client's browser receives the response and renders the page.
Subsequent Requests
- When the same PHP script is requested again, the server first checks if the compiled opcode for that script is already in OPcache.
- If the compiled opcode is found in OPcache (a cache hit), the server uses this cached version instead of recompiling the script. This step significantly speeds up the process.
- The cached opcode is executed to fulfill the request.
- the output is sent to the client's browser, just as in the first request.
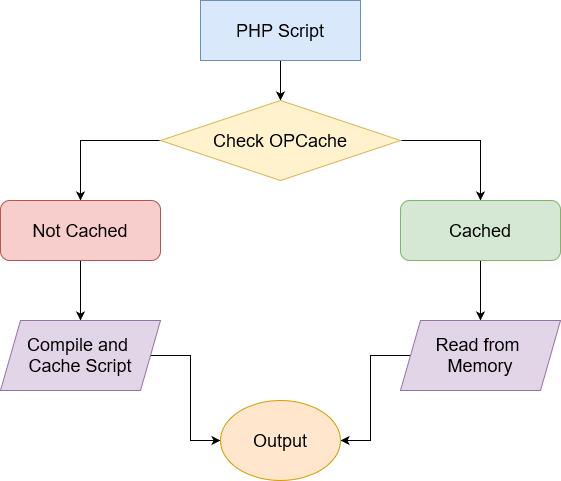
Think of OPcache as a shortcut that helps PHP serve your web pages more quickly by remembering the work it has already done.
Enabling OPCache
Enabling OPcache in PHP is usually straightforward. Believe it or not, it's already enabled in most PHP installations. If your server is using PHP 5.5 or higher, chances are it's enabled. You can verify this by running the following code:
phpinfo();
On the page produced by the function, search for the following: Zend OPcache
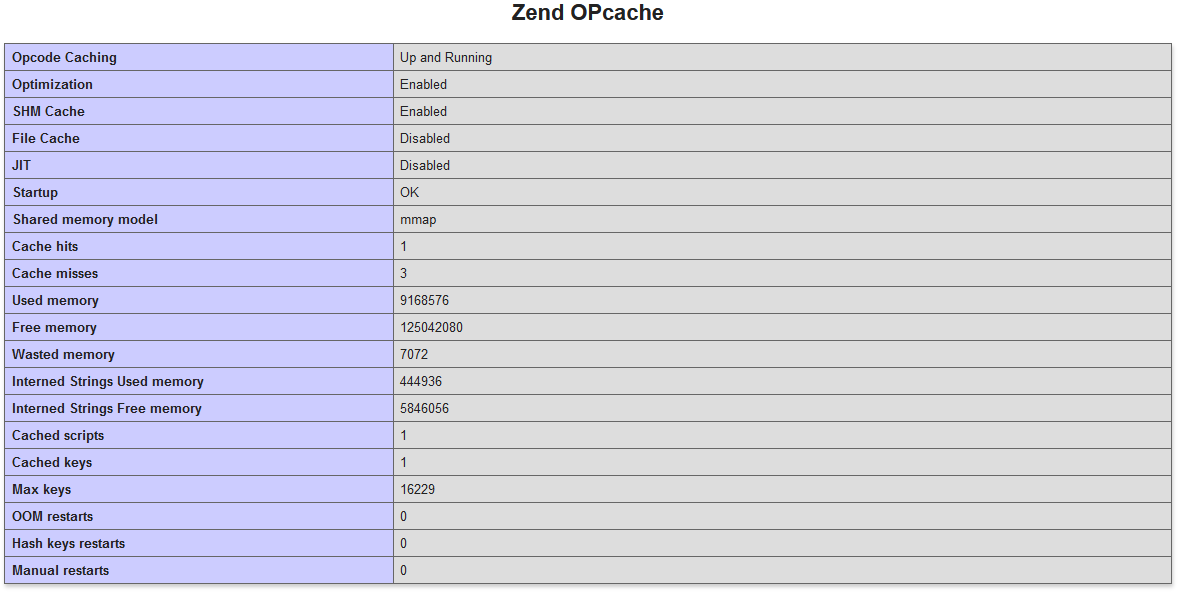
Under this section, the Opcode Caching
setting will be set to Up and Running
like in the image above.
Configuring Opcache
The OPcache extension can be configured through the php.ini
configuration file. You can enable the extension with the following line:
zend_extension=opcache.so
Note: The above line may vary depending on your operating system and PHP installation.
You can finetune opcache further with the following settings:
Setting | Description | Example Value |
---|---|---|
opcache.enable | Enables or disables OPcache. | 1 |
opcache.memory_consumption | Sets the amount of memory used by OPcache (in MB). | 128 |
opcache.interned_strings_buffer | Amount of memory used for interned strings, in MB. | 8 |
opcache.max_accelerated_files | Maximum number of files that can be accelerated by OPcache. | 4000 |
opcache.revalidate_freq | Frequency to check for file changes (in seconds). | 60 |
opcache.fast_shutdown | Enables a faster shutdown sequence for more performance. | 1 |
opcache.validate_timestamps | Validates timestamps of cached files. | 1 |
Here's an example of what the php.ini
file could look like:
zend_extension=opcache.so ; Path might vary based on your system
opcache.enable=1
opcache.memory_consumption=128
opcache.interned_strings_buffer=8
opcache.max_accelerated_files=4000
opcache.revalidate_freq=60
opcache.fast_shutdown=1
Please be aware that these settings will differ from host to host. You should configure these settings according to your application's needs.
Key Takeaways
- Caching is the process of storing previously computed or retrieved data so that future requests for the same data can be served more quickly.
- PHP uses caching mechanisms like OPcache to improve performance by storing precompiled script bytecode in the server's memory.
- OPcache is an opcode cache used in PHP that compiles PHP scripts into opcode once and reuses it for subsequent requests, reducing the need for recompilation.
- OPcache is enabled by modifying the
php.ini
file and adding or uncommenting the necessary lines related to OPcache. Then, the web server must be restarted.