Array Mutation Functions
Arrays can be mutated with a few handy functions offered by PHP. Here are some of the following:
array_push()
: This function is used to add one or more elements to the end of an array.array_unshift()
: This function is used to add one or more elements to the beginning of an array.array_shift()
: This function removes the first element from an array and returns that element.array_pop()
: This function removes the last element from an array and returns that element.array_unique()
: This function removes duplicate values from an array.array_merge()
: This function merges one or more arrays into one array.unset()
: This function is used to destroy specified variables. When used with an array, it can remove an element.
Let's go through each of them.
Adding Items to an Array
Two of the most common functions for adding items to an array are the functions array_push()
and array_unshift()
.
array_push()
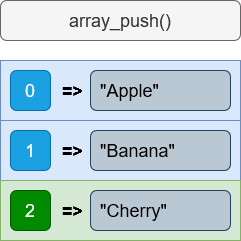
array_push()
is used to add one or more elements to the end of an array. It's like pushing a new item onto a stack of items. Here's how it works:
$fruits = array("Apple", "Banana"); // Let's start with an array of fruits.
array_push($fruits, "Cherry"); // Now, we use array_push to add "Cherry" to the end.
Now, our $fruits
array looks like this:
Array ( [0] => Apple [1] => Banana [2] => Cherry ).
You can also add multiple items at once:
array_push($fruits, "Date", "Elderberry");
This will add both "Date"
and "Elderberry"
to the end of the array.
array_unshift()
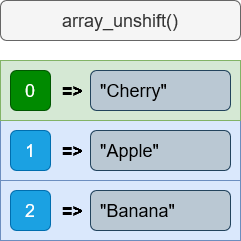
array_unshift()
is used to add one or more elements to the beginning of an array. It's like someone cutting in line - they go straight to the front. Here's how it works:
$fruits = array("Apple", "Banana"); // Again, we start with an array of fruits.
array_unshift($fruits, "Cherry"); // We use array_unshift to add "Cherry" to the beginning.
Now, our $fruits
array looks like this:
Array ( [0] => Cherry [1] => Apple [2] => Banana ).
And just like array_push()
, you can add multiple items at once:
array_unshift($fruits, "Date", "Elderberry");
This will add "Date"
and "Elderberry"
to the beginning of the array.
In summary, array_push()
and array_unshift()
are used to add items to an array. The difference is where they add the items - array_push()
adds to the end, and array_unshift()
adds to the beginning. They both change the original array directly, so be aware of that when using them.
Removing items from an array
There are three main functions for removing items from an array.
array_shift()
The array_shift()
function in PHP is used to remove the first element from an array and return that element. This operation changes the original array by reducing its length by one and shifting the indices of the remaining elements down by one. In other words, after array_shift()
is called, the second element of the array becomes the first, the third becomes the second, and so on.
In this example, array_shift($fruits)
removes the first element ("Apple"
) from the $fruits
array and returns that element. After the shift, the $fruits
array contains only "Banana"
and "Cherry"
.
This function is particularly useful when you're using an array as a queue (first-in, first-out), and you want to retrieve and remove the first element added to the array.
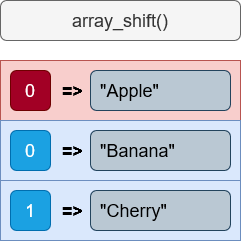
$fruits = array("Apple", "Banana", "Cherry");
$removedFruit = array_shift($fruits);
// $removedFruit now holds "Apple"
// $fruits is now array("Banana", "Cherry")
array_pop()
The array_pop()
function in PHP is used to remove the last element from an array and return that element. This function reduces the size of the array by one. It's the opposite of array_push()
, which adds an element to the end of an array.
In this example, array_pop($fruits)
removes the last element ("Cherry"
) from the $fruits
array and returns that element. After the operation, the $fruits
array contains only "Apple"
and "Banana"
.
This function is especially useful when you're using an array as a stack (last-in, first-out) and you want to retrieve and remove the most recently added element.
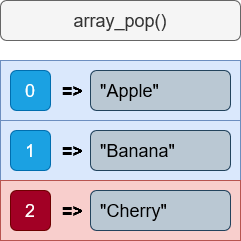
$fruits = array("Apple", "Banana", "Cherry");
$removedFruit = array_pop($fruits);
// $removedFruit now holds "Cherry"
// $fruits is now array("Apple", "Banana")
array_unique()
The array_unique()
function in PHP is used to remove duplicate values from an array. It returns a new array with all the duplicate values removed. The keys are preserved in the returned array, so you may see gaps in the numeric keys.
In this example, array_unique($fruits)
removes the duplicate entries ("Apple"
and "Banana"
) from the $fruits
array and returns an array that contains only unique values.
It's important to note that array_unique()
compares elements as strings, which can lead to unexpected results when dealing with numeric values. Look at the second code example. The strings '3'
and '03'
will be considered the same, and one will be removed.
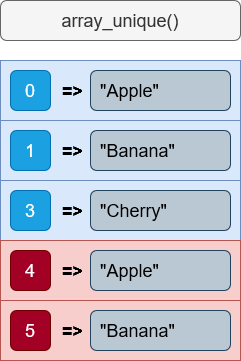
$fruits = array("Apple", "Banana", "Cherry", "Apple", "Banana");
$uniqueFruits = array_unique($fruits);
// $uniqueFruits now holds array("Apple", "Banana", "Cherry")
$numbers = array(3, '3', '03');
$uniqueNumbers = array_unique($numbers);
// $uniqueNumbers now holds array(3, '03')
Alternative function to remove items
There's another function available for removing items from an array called unset()
. The unset()
function in PHP is used to destroy or remove a variable or an array element. When used with an array, you can remove an element by specifying its key.
$fruits = array("Apple", "Banana", "Cherry");
unset($fruits[1]); // Removes the element at index 1 ("Banana")
// $fruits is now array("Apple", "Cherry")
In this example, unset($fruits[1])
removes the element at index 1
("Banana"
) from the $fruits
array.
The key difference between unset()
and the other array removal functions like array_pop()
and array_shift()
is that unset()
allows you to remove an element at any position in the array, not just the beginning or end. Also, unset()
doesn't reindex the array, so there will be a gap in the indices after the operation.
Another point to note is that unset()
does not return the removed element, unlike array_pop()
and array_shift()
. Once an element is removed using unset()
, it is lost unless you've stored it somewhere else beforehand.
Merging Arrays
The array_merge()
function in PHP is used to merge two or more arrays together into a single array. This function returns a new array that contains all the elements of the input arrays.
$array1 = array("Apple", "Banana");
$array2 = array("Cherry", "Date");
$result = array_merge($array1, $array2);
// $result now holds array("Apple", "Banana", "Cherry", "Date")
In this example, array_merge($array1, $array2)
merges the two arrays $array1
and $array2
together and returns an array that contains all the elements from both input arrays.
You can merge more than two arrays at once:
$array1 = array("Apple", "Banana");
$array2 = array("Cherry", "Date");
$array3 = array("Elderberry", "Fig");
$result = array_merge($array1, $array2, $array3);
// $result now holds array("Apple", "Banana", "Cherry", "Date", "Elderberry", "Fig")
It's important to note that if the input arrays have the same string keys, then the later value will overwrite the earlier value:
$array1 = array("fruit" => "Apple", "color" => "Red");
$array2 = array("fruit" => "Banana", "taste" => "Sweet");
$result = array_merge($array1, $array2);
// $result now holds array("fruit" => "Banana", "color" => "Red", "taste" => "Sweet")
In this case, both $array1
and $array2
have the key "fruit"
. In the merged array, "fruit" => "Banana"
from $array2 overwrites "fruit" => "Apple"
from $array1
.
Key Takeaways
array_push()
: Adds one or more elements to the end of an array. Mutates the original array and returns the new number of elements.array_unshift()
: Adds one or more elements to the beginning of an array. Mutates the original array and returns the new number of elements.array_shift()
: Removes the first element from an array and returns it. Mutates the originalarray_pop()
: Removes the last element from an array and returns it. Mutates the original array.array_unique()
: Removes duplicate values from an array. Returns a new array with unique values, original array remains unchanged.unset()
: Destroys or removes a variable or an array element. Does not return the removed element and does not reindex the array.array_merge()
: Merges two or more arrays together into a single array. Returns a new array that contains all the elements of the input arrays.