Abstraction
Abstraction is one of the four pillars of Object-Oriented Programming (OOP) - the other three being encapsulation, inheritance, and polymorphism.
In simple terms, abstraction means dealing with the level of detail that is most appropriate to a task. It's about hiding the complexity and only showing the essential features of an object or a system.
Imagine you're using a microwave. To heat your food, you don't need to understand how electromagnetic waves are generated or how they interact with your food. You just need to know how to set the timer and the power level. The microwave "abstracts away" the complex details and provides you with a simple interface: a few buttons and a display.
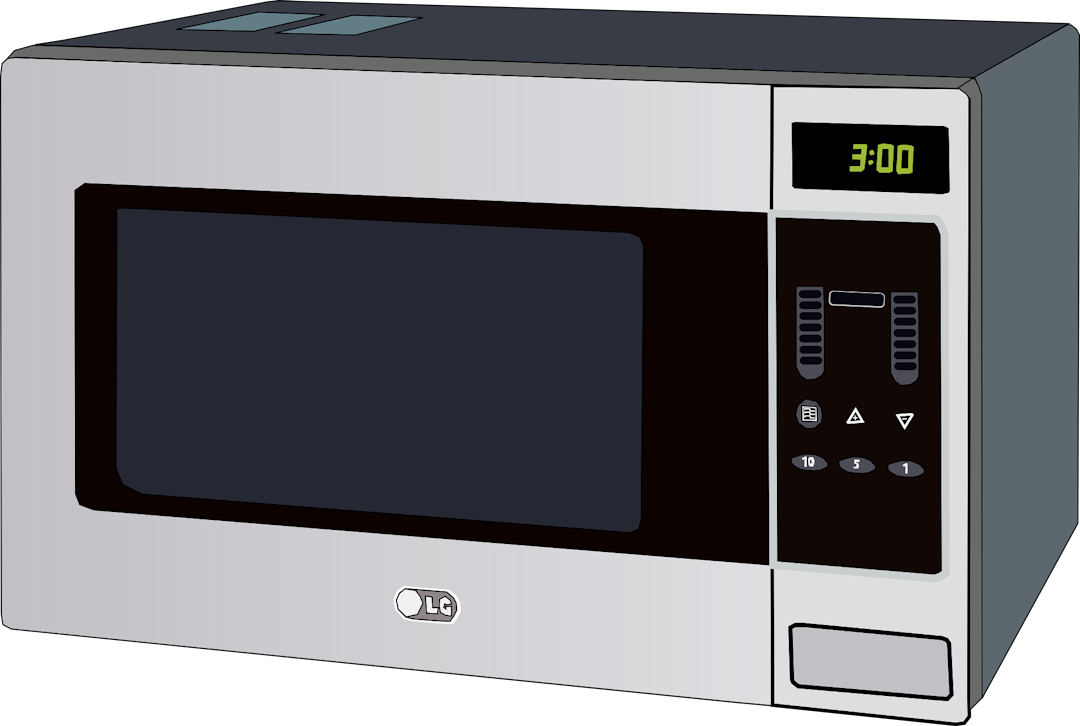
Applying the abstraction principle
In PHP and other OOP languages, an abstract class can be used to represent such an abstraction. An abstract class is a class that cannot be instantiated on its own and is designed to be subclassed by other classes. It might contain one or more abstract methods, which are declared but not implemented in the abstract class. Any class that extends the abstract class is responsible for implementing these methods.
We've already learned about abstract classes. If you need a refresher, check out this lesson.
Why is abstraction important?
Abstraction is essential because it allows you to isolate how an object performs an action from the actual implementation of how it performs that action. This lets you write code that can work with any object that implements the interface, regardless of the underlying details.
It makes complex systems easier to handle by breaking them down into smaller, more manageable parts. It also promotes code reuse and helps in hiding the complexities, enabling us to focus on the essential features of an object.
Abstract classes vs Interfaces
Abstract classes and interfaces are both ways to enforce certain structures in your code, but they work a little differently.
Interfaces are like contracts or promises. When a class implements an interface, it promises to provide certain methods (functions). However, the interface itself doesn't provide any code for these methods; it just specifies what the methods should be. It's up to the class that implements the interface to provide the actual code.
Abstract classes, on the other hand, are like "half-finished" classes. They can provide some methods with full code (just like a regular class), but they can also have abstract methods. Abstract methods, like interface methods, don't have any code in the abstract class; any class that extends the abstract class has to provide the code.
Here are a few key differences:
Abstract Class | Interface | |
---|---|---|
Definition | A class that cannot be instantiated and may contain implementations and abstract methods. | A contract that defines a set of methods that a class must implement. |
Instantiation | Cannot be instantiated directly. | Cannot be instantiated directly. |
Inheritance | Can extend only one abstract or concrete class. | Can implement multiple interfaces. |
Method Definitions | Can contain both implemented methods and abstract methods. | Contains method signatures only, without any implementations. |
Method Implementation | Abstract methods must be implemented by the extending class. | All methods defined in the interface must be implemented. |
Access Modifiers | Can have methods with public, protected, or private access modifiers. | Methods are implicitly public in the interface. |
Properties | Can have properties with any access modifiers. | Cannot have properties. |
Common Use Case | Used when creating a base class with some common functionality. | Used when defining a contract that multiple classes must adhere to. |
Using abstract classes and interfaces
It's completely acceptable to use both abstract classes and interfaces at the same time. For instance, imagine a zoo. All animals eat, so we can have an abstract class Animal
with a method eat()
. But not all animals fly. So we could have an interface CanFly
with a method fly()
, and only animals like birds would implement CanFly
.
Here's how you might write that:
abstract class Animal {
public function eat() {
// Code for eating.
}
}
interface CanFly {
public function fly();
}
class Bird extends Animal implements CanFly {
public function fly() {
// Code for flying.
}
}
In this example, Bird
is an Animal
, so it inherits the eat()
method. And because it's a bird and can fly, it also implements CanFly
and provides a fly()
method.
Key Takeaways
- Abstraction is about simplifying complex systems by hiding unnecessary details and exposing only what's necessary. Just like using a remote control without knowing its internal workings.
- In PHP, abstraction is achieved through 'abstract classes'. These classes cannot be instantiated directly and are meant to be extended by other classes.